Fix ‘custom_tags’ is not a registered tag library: must be one of: error
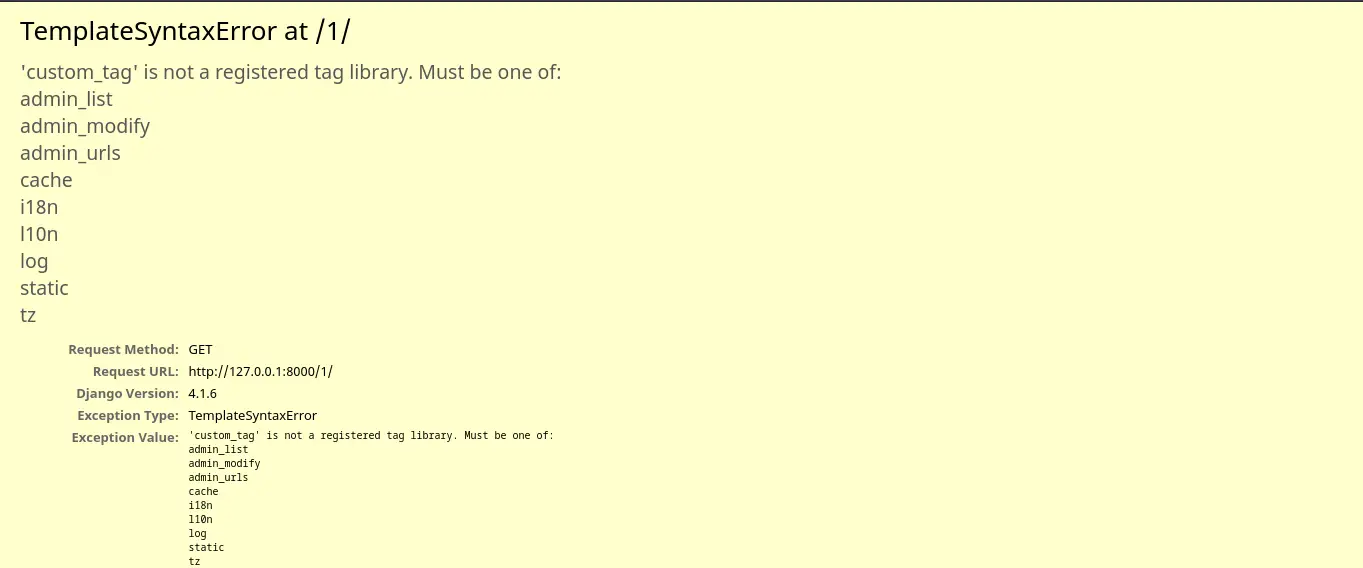
As a Django web dev, you know that creating versatile and effective web applications is a great flex. However, that flex can be extinguished mid-journey when you have errors like ‘custom_tags’ is not a registered tag library: must be one of: … that keeps you from completing your project in time.
Worry no more. I got you, mate.
As you know, using custom tags is a great way to add extra functionality and structuring to your Django templates. They allow you to add Python-like features such as looping and conditional statements inside your normal .html files.
When creating these custom template tags, you are much more likely to face the ‘custom_tags’ is not a registered tag library error. This can be frustrating.
In this article, I’ll show you how to resolve the error, and probably help you understand why the errors occur and the best practices to follow when creating custom template tags.
What is “‘custom_tags’ is not a registered tag library: must be one of: admin_list admin_modify admin_urls cache i18n l10n log static tz” error?
The “custom_tags is not a registered tag library: must be one of:” is an error message encountered in Django when a custom tag library is not recognized as a valid tag library. The error occurs if the module containing the tag library is not included in the INSTALLED_APPS list or if the conventions for creating valid tag libraries are not followed.
Django is known for its clean and efficient design. One of the key features that Django provides is the ability to create custom tags, which are functions that can be used to add custom functionality to your templates.
having custom tags can make your Django templates more extensive, readable, and easier to maintain, as well as make it easier to reuse code in different parts of your application.
However, creating custom tags in Django requires careful consideration of conventions to avoid getting exceptions such as “custom_tags is not a registered tag library”. If the tag library is not properly registered or configured, you may end up having the error message “custom_tags is not a registered tag library: must be one of: admin_list admin_modify admin_urls cache i18n l10n log static tz”.
This error message indicates that the tag library is not recognized as a valid tag library by Django, and as a result, the custom tags defined in the library cannot be used in your templates.
Causes of ‘custom_tags’ is not a registered tag library: must be one of: error
Custom tags are powerful features you can implement in your Django templates. When creating custom tags you avoid making these mistakes so that you do not get the 'custom_tags' is not a registered tag library: must be one of:
exception in your web pages:
- Missing to include the app with the custom tag library module in the
INSTALLED_APPS
list in the settings.py file. - The module-level variable named ‘register’ is not defined as an instance of the Library class.
- The custom template tags defined in your tags module are not properly registered using the correct syntax that follows:
register.tag()
method. - Incorrectly referencing the tag library module name.
- Creating or specifying the tag library module in the wrong location.
- Failing to register the Django app containing custom template tags in the
INSTALLED_APPS
list in the settings.py file. - Not loading the module with the custom tags into the template where the custom template tags are used.
- The custom template tags are being used in a template file that is not associated with the same app as the tag library.
- Incorrect use of the Library class in your custom tags module.
- Missing to place custom tag modules inside the Django app-level directory.
- Missing the __init__.py file in the templatetags directory.
- Not restarting the Django development server after making changes to your custom tags code.
- Missing to import the template module from Django (
from django import template
) in your tag library module.
How to fix ‘custom_tags’ is not a registered tag library: must be one of: error
When creating custom template tags to enhance the functionality and readability of your Django templates, you may encounter the error message “custom_tags is not a registered tag library:”. This error indicates that there is a problem with the way your custom tags are set up in your application.
Let’s explore the different solutions for each cause identified above to help you resolve the error based on what you are missing.
Ways to fix the “custom_tags is not a registered tag library: must be one of: ” exception
- Include the Django app with the custom tags library in INSTALLED_APPS
- Use the correct syntax for writing valid custom tags. use the @register.tag decorator as an instance of the Library class.
- Properly register custom tags using the register.tag() method
- Reference tag library module name correctly
- Specify the tag library module from the correct location
- Load custom tag module into the template using template tags
- Use custom tags in templates associated with the same app
- Correctly use the Library class when defining the custom tags module
- Place custom tag modules inside the app-level directory or the project-level directory, and not the root directory.
- Include the __init__.py file in your templatetags directory
- Restart the Django development server after making changes to your custom tags code.
- Import the ‘template’ module from Django using the syntax:
from django import template
Here are the detailed solutions for the custom_tags is not a registered tag library.
Include the Django app with the custom tags library in INSTALLED_APPS
To include the Django application with the custom tags library in the list of INSTALLED_APPS, you need to make a simple change to the settings.py file in your Django project.
Here are the steps to include the app with the custom tags library in INSTALLED_APPS:
Step 1: Open the settings.py file and locate the INSTALLED_APPS list
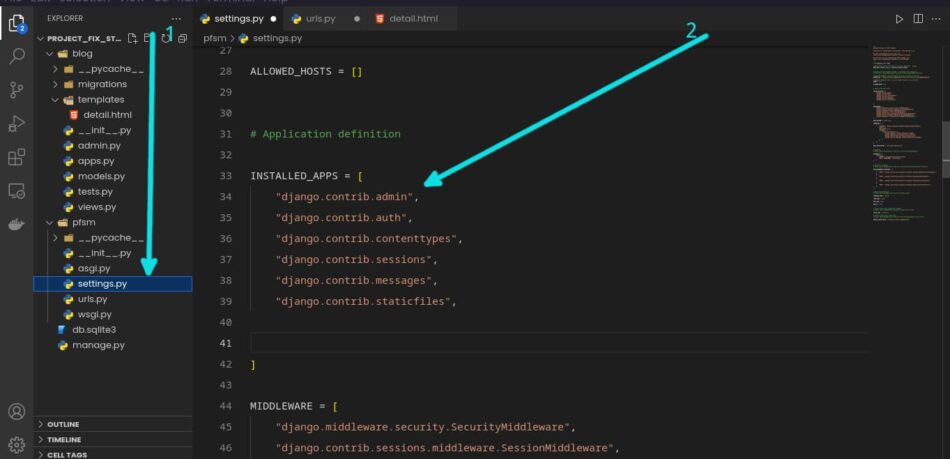
Step 2: Add the app with the custom tags library
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
# new line
'name_of_the_app_here',
]
The name of the app should be exactly the same as the name of the directory containing the app’s code.
Step 3: Save the file and restart Django development server
After you have added the app with the custom tags library to the INSTALLED_APPS list, save the changes to the file and close it.
To ensure that the changes to the settings.py file take effect, you will need to restart the Django development server. This can be done by running the command python manage.py runserver
in the terminal.
python manage.py runserver
You should now have included the Django app with the custom tags library in the INSTALLED_APPS list, resolving any errors related to the custom tags not being registered.
Use the correct syntax for writing valid custom tags. use the @register.tag decorator as an instance of the Library class.
To write valid custom tags in Django, you must use the correct syntax and make sure that the custom tags are written correctly. Here are the simple steps to follow to create the correct syntax for custom tags:
1. Import the template module from Django
from django import template
2. Define a module-level variable named register
that is an instance of the Library class
register = template.Library()
3. Create each custom tag using the def keyword and use @register.tag decorator
@register.tag
def my_custom_tag(parser, token):
...
4. Use your new valid custom tag in your Django templates
Properly register custom tags using the register.tag() method
You must properly register a custom tag using the @register.tag decorator, which allows the custom tag to be used in your Django templates.
Reference the tag library module name correctly
Make sure that the name you use for the module in your templates matches the name of the actual module that you have created to store your custom tags.
For example, if your tag library module is named “my_custom_tags.py”, then you must reference it as “my_custom_tags” in your templates, rather than using a different name like “my_tags”.
Django uses the module name you provide in your templates to look up the actual module and check if it is a valid tag library. If the name you provide doesn’t match the actual name of the module, Django will not be able to find the module and will raise an error.
Specify the tag library module from the correct location
It is important to specify the location of the tag library module correctly. This is because Django uses the specified location to search for the custom tags when they are used in the templates. If the location of the tag library module is incorrect, Django will not be able to find the custom tags, and an error will occur.
To ensure that the tag library module is specified correctly, make sure that the module is located in the correct directory structure within your Django project. Typically, custom tag library modules must be created inside an app-level directory in a directory called templatetags
.
Load the custom tag module into the template using your custom template tags
To load a custom tag module into a template in Django, you need to use the `{% load … %} template tag. The {% load … %} template tag takes an additional argument, which is the name of the module containing your custom tags.
So, in your template file, add the following line at the top. Remember to use the correct name of the module containing the custom tags.
{% load my_custom_tags_module_name %}
Here, my_custom_tags_module_name
is the name of the custom tag library module. Once the custom tag library module is loaded, you can use the custom tags in your template. To use a custom tag, simply include the tag name within double curly braces {{ }}
.
{{ my_tag }}
Use custom tags in templates associated with the same app
When using custom tags in Django templates, ensure that the templates are associated with the same app as the tag library. This helps to avoid the error message, “custom_tags’ is not a registered tag library: must be one of: admin_list admin_modify admin_urls cache i18n l10n log static tz”.
Here are some simple steps to follow:
I. Create app-level templates directory
Create a templates directory within the app, and make sure it’s defined in the DIRS option in the TEMPLATES setting in the settings.py file.
mkdir your_app_name/templates
II. Create your template file inside the app-level templates directory
Place the template file that will use the custom tags in the templates directory associated with the app.
III. Load the custom tag library module in the template file using the ‘{% load %}’ template tag
Use the custom tags in your template file as needed.
Correctly use the Library class when defining the custom tags module
To correctly use the Library class when defining the custom tags module, follow these simple steps:
Step 1: Import the template module from Django
In your custom tags module,
from django import template
Step 2: Create an instance of the Library class
Create an instance of the Library class by defining the module-level variable named ‘register’.
register = template.Library()
Use the @register.tag decorator to register your custom tags with the library.
@register.tag
def your_custom_tag():
...
By following these steps, you can correctly use the Library class when defining your custom tags module, which is essential for avoiding the “custom_tags is not a registered tag library: must be one of” error.
Place custom tag modules inside the app-level directory or the project-level directory and not the root directory
Placing custom tag modules inside the app-level directory or project-level directory is important to ensure that your custom tags can be correctly imported and recognized by Django across your web application. You need to make sure that your custom tags module is located inside either the app-level directory or the project-level directory and not the root directory.
Here’s an example of what your file structure might look like:
Project_Name
- App_Name
- templatetags
- custom_tags.py
- __init__.py
- models.py
- templatetags
- Project_Name
- templatetags
- another_custom_tags.py
- __init__.py
- settings.py
- templatetags
You can place your custom template tags module inside the project-level directory if you want to make it available for use in all the apps in your project. Just make sure to create a “templatetags” directory inside the project-level directory and place the custom tags module inside that directory.
Include the __init__.py file in your templatetags directory
Make sure that the tag library module is placed inside the Django app-level directory and that it includes an __init__.py
file in the templatetags directory.
# Create the templatetags directory
mkdir app_name/templatetags
# Create __init__.py file inside the templatetags directory
touch app_name/templatetags/__init__.py
Restart the Django development server after making changes to your custom tags code
It is a good practice to restart the Django development server after making changes to the custom tags code. The changes you made to your custom tags may not take effect until you restart the development server.
Here is how you can restart the development server:
Stop the running server by either pressing “CTRL + C” in the terminal where the server is running.
Re-run the development server by running the following command in the terminal:
python manage.py runserver
Import the ‘template’ module from Django using the syntax: from django import template
Import the ‘template’ module, so that you’ll have access to the Library
class and the register
object, which are both essential for creating custom tags in Django. The Library
class allows you to create a tag library, which is a collection of custom tags, and the register
object allows you to register the custom tags you’ve created in the tag library.
So, at the top of each custom template tags module, add:
from django import template
To resolve the error, the module containing the tag library must be included in the INSTALLED_APPS list and the conventions for creating valid tag libraries must be followed.
Conclusion
The ‘custom_tags’ is not a registered tag library error is a common exception encountered when working with Django’s template system. The error occurs when the custom tag library has not been correctly set up or registered.
In order to fix this error, it’s important to make sure that:
- The Django app or third-party package containing the custom tag library is included in the INSTALLED_APPS list in the settings.py file.
- The custom tags are properly defined using the correct syntax and should be loaded into the template where they will be used.
- The custom tags modules are placed in the correct location and referenced correctly.
- The Library class is correctly used when defining the custom tags module.
- Restarting the Django development server after making changes to the custom tags code