How to add two values in Django templates
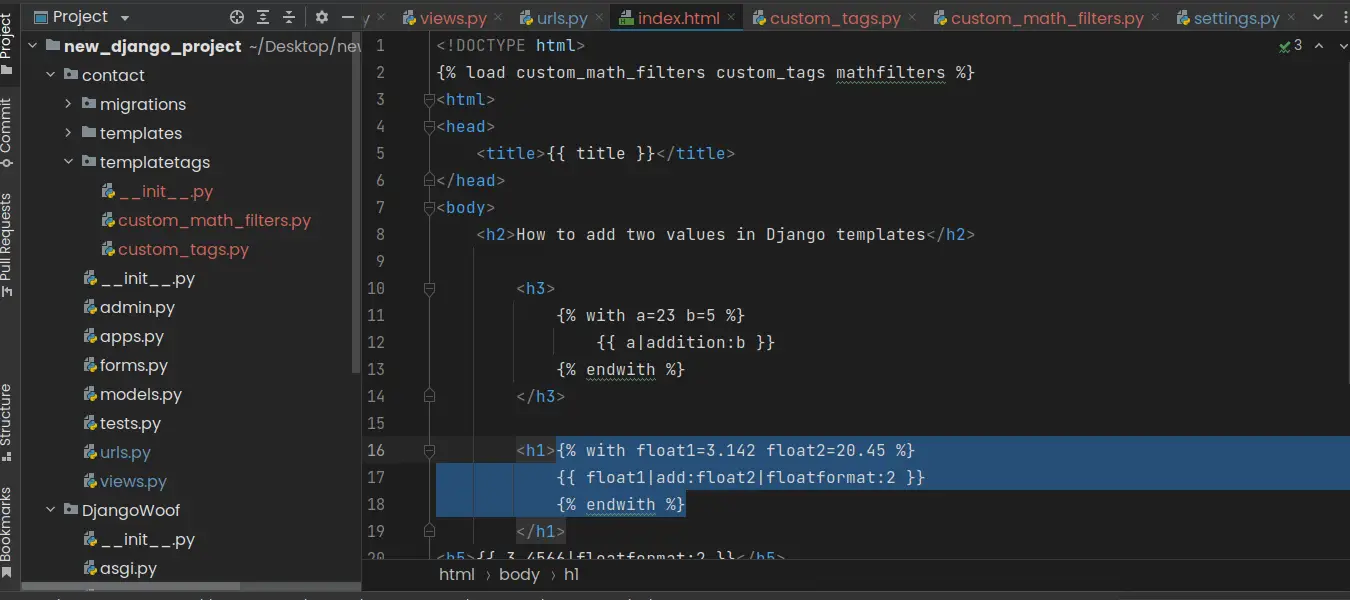
With Django templates, you have the freedom to do anything you can think of. For example, if you wish to add two values/variables in a template, you can do so using Django’s default template filters. Besides, you can also create your own custom tag to add two values.
The possibilities are endless. Whether you want to calculate total price based on user selection, adding up student’s scores, add basic numbers, Django provides you with that flexibility.
In this article, we’ll explore various approach to adding two values in Django templates using built-in filters and custom template tags.
You can add two values in Django templates using the built-in
add
filter that takes two arguments and returns their sum. The correct syntax is {{ value1|add:value2 }}. Alternatively, you can use django-mathfilters package or your own custom template tags and filters, to implement more complex arithmetic operations in Django templates.
Let’s see in detail how to add two variables in Django templates using each approach.
How to add two values in Django templates
In general, there are different approaches you can use to add two values in Django templates. Among these approaches are:
- Django built-in
add
filter - Custom template filter
- Custom template tag
- Using
django-mathfilters
library
Let’s see how each approach works!
1. Add two values in Django templates using built-in add
template filter
Django templates provide a powerful set of built-in filters to manipulate the data before rendering it. One of them is the add
template filter that adds two values and returns the result.
To use the add
filter in a Django template, you need to first define the two values you want to add. You can use variables or literals for this. Once you have the values, you can apply the add
filter to them using the |
(pipe) symbol.
For example, if you have two variables a
and b
that contain the values 10 and 5, respectively, you can add them in a template as follows:
{{ a|add:b }}
This will output the result of adding a
and b
, which is 15 in this case.
How to assign variables some values in Django templates?
To assign values in a Django template, you can use the {% with %} template tag. For our example, you can assign the values of and b as follows:
{% with a=3 b=5 %}
{{ a|add:b }}
{% endwith %}
You can also add literals directly in the template using the add
filter. For example, to add the values 2 and 3, you can use:
{{ 2|add:3 }}
This will output the result of adding 2 and 3, which is 5.
It’s important to note that the
add
filter only works with values that can be added mathematically. If you try to add two strings or two lists using theadd
filter, you will get an error.
How to add more than two values in Django templates
If you want to add more than two values in a Django template using the add
filter, you can chain multiple filters together using the |
symbol.
For example, let’s say you have three variables a
, b
, and c
that contain the values 25, 50, and 100, respectively, and you want to add them together in a template. You can do this by applying the add
filter twice, like so:
{% with a=25 b=50 c=100 %}
{{ a|add:b|add:c }}
{% endwith %}
This will first add a
and b
, which results in 75, and then add the result with c
, which gives a final result of 175.
You can chain as many add
filters together as you need to add more values. However, it’s important to note that the order of operations matters.
In the above example, if you change the order of the filters like this:
{{ a|add:c|add:b }}
You will get same result of 175 but with a different order of operation because a
is first added with c
to get 125, and then b
is added to the result to get 175.
With the default add
template filter, you can do pretty much any numerical addition. The addition of string values is not supported in Django templates using the add
template filter.
2. Add two variables using a custom template filter
Another approach to adding two values in Django templates is to use your own custom template filter.
Here is a step-by-step process that you can follow to create your own custom template filter for adding two variables with it.
Step 1: Identify the Django app you wish to create the template filter in and create templatetags
folder
You should create your new custom template filter Python file in a Django app that is recognized in your Django project. That means, the app should be listed in the INSTALLED_APPS variable in the main project’s setting.py file.
Inside your app, create a new directory called templatetags
. This directory is used to store all your custom template tags and filters used for that particular app or whole project.
If you face some issues with your custom template tags in your templates, make sure you have __init__.py file inside the templatetags directory.
__init__.py should be an empty file.
Step 2: Create a new Python file to hold the custom filter code
Inside your Django app templatetags directory, create a new Python file giving it a descriptive name that follows its intended purpose.
Let’s say my Django app is called blog
, then inside the blog directory, I should create a new Python file called: custom_math_filters.py
Name the file any name you want and you will remember.
Step 3: Import the necessary decorators and classes for registering a new template filter
Inside your new Python file, import the register
decorator and template.Library() class from the Django package. You can do so like this:
from django import template
register = template.Library()
Step 4: Define the function for accepting two numbers and returning their sum
For our case, we are creating a new Django template filter for adding two numbers. So, we define a new function called addition
or add_values
or any name you wish to have for your filter.
@register.filter
def addition(a, b):
return a + b
Note: The function should be decorated with the register.filter decorator to be recognized as a new template filter.
The function name (addition
) will be the name of our custom template filter.
Save the custom_math_filters.py file.
Step 5: Load the custom template filter in your template file
In your Django template, load your custom filter by adding this line at the top of your template file:
{% load custom_math_filters %}
load
is a builtin django template tag that makes custom template tags available for use in a particular template.In this case, the load make all the custom filters declared inside our custom_math_filters available for use in a template file.
Step 6: Use your new custom template filter to add two numbers
You can now use the add_values
filter in your template like this:
{% with a=25 b=75 %}
{{ a|addition:b }}
{% endwith %}
The a
and b
values will be passed as arguments to the add_values
function in custom_math_filters.py
, and the result will be returned and rendered in your template.
In this case, you should get 100.
3. How to add two values in Django templates using custom template tag
You can also use custom template tags to add two or more values in Django templates. If you wish to create an addition template tag to add two numbers, you can do so by following these steps:
I. Create a new templatetags
directory inside your Django app directory
To create a custom template tag that adds two values together in Django, the first step is to create a new templatetags
directory within your app directory.
This directory will contain the Python module that defines your custom tag.
mkdir app_name/templatetags
# For a Django app called, blog, use
mkdir blog/templatetags
II. Inside the templatetags
folder create __init__.py file and your new Python file to hold your tag logic
Inside the templatetags
directory, create an empty __init__.py
file and the Python module that will hold the logic for your custom tag.
This module will be imported by Django when it loads the template tags for your app.
cd app_name/
touch templatetags/__init__.py
touch templatetags/custom_tags.py
III. Inside the new Python file, import the necessary decorators and classes
In the new Python module, import the necessary decorators and classes that you will need to define your custom tag.
These include the template.Library
class and the register
decorator that you will use to register your custom tag with Django’s template system.
IV. Define a new function that will accept two values and return their sum
Define a new function that will accept two values as its arguments and return their sum.
This function will contain the logic for your custom tag. You can use any Python code you like inside this function to perform the addition.
from django import template
register = template.Library()
def add_values(a, b):
return a + b
In this example, the register
decorator tells Django that this function is a template tag that can be used in templates.
The add_values
function takes two arguments, a
and b
, and returns their sum.
V. Decorate the function with @register.simple_tag
decorator
Decorate your custom tag function with the @register.simple_tag
decorator. This decorator tells Django that your function is a simple template tag that doesn’t require a closing tag.
@register.simple_tag
def add_values(a, b):
return a + b
VI. In your Django templates, load the custom template tag module and use the tag to add two values
In your Django templates, load the custom template tag module using the {% load %}
tag.
{% load custom_tags %}
This makes your custom tag available for use in your templates.
You can then use your custom tag to add two values together by passing them as arguments to the tag.
The sum of 2 and 3 is {% add_values 2 3 %}.
In this example, the load
template tag tells Django to load the custom_tags
module so that the add_values
tag can be used.
The add_values
tag takes two arguments, 2
and 3
, and returns their sum, which is displayed using the {
% add_values … %} template tag.
How to add more than two values using a custom template tag
Sometimes, you may want to add more than two numbers in a Django template. To do that, follow these detailed steps:
If you have been following the previous steps, skip the steps that you have already accomplished.
a) Create a new directory inside your Django app directory called templatetags
.
mkdir app_name/templatetags
b) Create __init__.py and template tag module files
Inside the templatetags
directory, create an empty file called __init__.py
to make it a Python package. This file tells Python that the templatetags
directory is a package that can be imported as a module.
touch templatetags/__init__.py
Also, create a new Python file to hold your custom tag logic. You can give this file any name you want, but it’s a good practice to give it a descriptive name that reflects the functionality of the tag.
touch templatetags/my_tags.py
c) Define your custom template logic by creating a function and decorating it with register.simple_tag
decorator
In the new Python file, import the necessary decorators and classes from Django’s template
module:
from django import template
register = template.Library()
Next, define a new function that will accept any number of values and return their sum.
This function should accept *args
as its argument, which means it can accept an arbitrary number of arguments.
Inside the function, use the built-in sum()
function to calculate the sum of all the arguments and return the result:
@register.simple_tag
def add_values(*args):
return sum(args)
In this example, we’ve used the simple_tag
decorator to register the function as a simple tag.
The *args
syntax in the function definition tells Python to collect any number of positional arguments passed to the function into a tuple named args
.
The sum()
function is then used to calculate the sum of all the values in args
.
d) Load the custom template tag in your Django template and use it to add arbitrary numbers
In your Django templates, load the custom tag by adding the {% load %}
statement at the top of the template, and use the tag to add any number of values:
{% load my_tags %}
{% add_values 2 4 6 8 10 %}
In this example, we’ve loaded the custom tag by calling {% load my_tags %}
, where my_tags
is the name of the Python file where we defined the add_values
function.
We’ve then called the add_values
tag and passed five integer values to it. The resulting sum (30
) will be output in the rendered template.
That’s it! You now have a custom template tag that can add any number of values in your Django templates.
4. How to add two values using django-mathfilters in templates
The next method that you can use to add two numbers in Django template is by installing the django-mathfilters package and using its already-declared template tags in your template files.
To start using django-mathfilters in your Django project, follow these steps:
Install django-mathfilters
package using pip
Install django-mathfilters package by running pip install django-mathfilters
in your command prompt or terminal.
Register django-mathfilters
to the list of INSTALLED_APPS
In your Django project settings.py file, add ‘mathfilters’ to the list of installed apps.
Load django-mathfilters
template tags in your template file
In your Django template, load the mathfilters using the {% load mathfilters %}
template tag at the top of the template.
Use django-mathfilters
addition
template filter to add two values
Use the mathfilters add template filter by passing two variables as arguments to it, like so: {{ variable1|addition:variable2 }}
.
Get the results!
The mathfilters add template filter will then add the two variables and output the result.
The mathfilters package provides many other useful mathematical filters that you can use in your Django templates, such as subtract (sub), multiply (mul), divide (div), absolute value (abs), modulo (mod), etc.
Using mathfilters not only saves you from writing custom template tags, but also provides a more concise and readable syntax for performing mathematical operations in your templates.
Best practices for adding variables in Django templates
- Avoid complex logic and calculations in templates
- Use context processors to prepare data then only present it in your templates
- Use custom template tags for more complex functionality
- Keep the logic of calculating and manipulating variables in the view or a separate function, and only pass the final values to the template.
- Use a clear and descriptive variable name to avoid confusion and make the code easier to understand.
- Avoid hardcoding values in the template, as it makes the template more difficult to maintain and update.
- Keep the HTML markup separate from the logic and data presentation in the template, by using template tags, filters, and variables to generate dynamic content.
Best approach to adding two values in Django templates
The best approach to adding two values in Django templates depends on the specific use case and your preference.
- Use a built-in template filter when you want a simple and straightforward way to add two values in Django templates.
- For more flexibility and customization, you can use custom template tags or filter to add two values in your Django templates.
- Finally, a third party can provide you with thoroughly tested customized, and flexible mathematical operations that you can use in your Django templates.
How to add floats in Django templates
You can easily add floats in your Django templates using the add
template filter provided by default in Django. To format the result, such as using two decimal places, you can use add filter with the floatformat filter together.
Here is a step-by-step process of adding floats in Django templates:
Step 1: Declare your variables in a context or using with
To declare variables in your Django templates, you can either use the context to hold the variables or use the with
template tag.
I’ll show you how to use the two approaches.
Approach one: Use the context
The context approach involves declaring your two float variables in your views.py file and passing the variables to the context that gets returned by the view. Thus, you can be able to access these variables in your templates.
Here’s how you would declare your variables in your views:
Define your view function in views.py
and declare two float variables:
# views.py
def my_view(request):
float1 = 3.25
float2 = 2.5
context = {'float1': float1, 'float2': float2}
return render(request, 'your_template.html', context)
By declaring your float1
and float2
variables in your view, you can be able to access their values in your template, your_template.html
.
You can read this article to learn more about how views work in relation to Django templates to create web pages.
Approach two: Use the with
tag
Alternatively, you can use the with
template tag to declare your variables inside the .html file.
Here’s how you use with tag to declare a variable in Django templates:
1. Identify the part of the template where you want to declare a new variable.
2. Use the with
tag to declare the new variable, followed by an equals sign and the expression that defines its value.
For example, if you want to declare a variable called float1
that holds a float, 3.412, you can do it like this:
{% with float1=3.412 %}
<p>The value of pi is: {{ float1 }}</p>
{% endwith %}
You can also declare multiple variables in your with
template tag by providing multiple variable declaration arguments to the with
tag. Here’s an example:
{% with float1=3.412 float2=10.24 %}
<p>The value of pi is: {{ float1 }}</p>
<p>Current bank balance: {{ float2 }}</p>
{% endwith %}
Step 2: Use the add
template filter to add two floats in your template file
After declaring your variables using either context variable or with template tag methods, you can add the two values using the add
template filter. The add filter, in Django templates, allows you to add two or more values. These values are supplied to the add
filter as arguments.
Here is how you can add 3.142 and 20.45 floats in your Django templates:
float1 = 3.142
float2 = 20.45
(Declared either using the approaches mentioned above)
In this example, I am using the template tag,
with
to declare the variables.
{% with float1=3.142 float2=20.45 %}
{{ float1|add:float2 }}
{% endwith %}
In this example, float1
and float2
are variables that contain float values. The add
template filter is used to add the two variables together, and the result is displayed in the template using {{ float1|add:float2 }}
.
Note that when working with floats, it’s important to keep in mind that they can sometimes result in rounding errors or other issues, so it’s a good idea to test and validate the results to ensure accuracy.
Step 3: Use the floatformat
filter to format the result
floatformat
is a built-in Django template filter that allows you to format a floating-point number to a specific number of decimal places in the template.
The syntax for using the floatformat
filter is as follows:
{{ value|floatformat:"n" }}
Here, value
is the floating-point number to be formatted, and n
is the number of decimal places to format the number to. If n
is not provided, floatformat
defaults to 1 decimal place.
For our example, we can format the result to get two decimal places by using the floatformat filter like this:
{% with float1=3.142 float2=20.45 %}
{{ float1|add:float2|floatformat:2 }}
{% endwith %}
Conclusion
In conclusion, adding two values in Django templates can be achieved using a variety of techniques, such as built-in template filters, custom template tags, third-party math filters, or even context processors.
It’s important to consider the best approach for your use case and ensure that you follow best practices for clean and maintainable code.
Separating presentation logic and business logic is crucial when dealing with adding variables in Django templates. It allows you to keep your code organized, easy to read and maintain, and also ensures that your presentation layer is decoupled from your business logic layer.
This can make it easier to make changes in the future and also makes your code more reusable.