How to use AND and OR operators in Django Templates
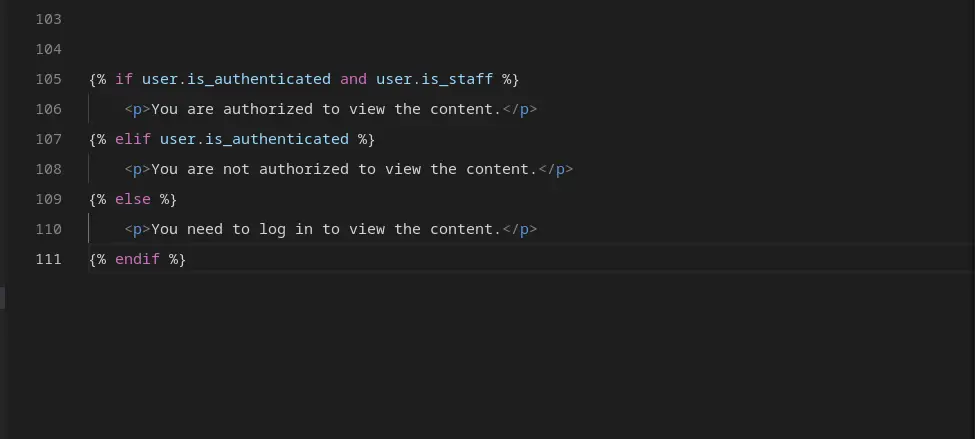
A few neons ago, while working on a project, I found myself staring at a particularly convoluted template that used a combination of if-else statements, and it was not immediately clear to me how to modify the code.
That’s when I learned about the logical operators AND and OR in Django Templates. These operators allow you to combine multiple conditions and evaluate whether a statement is true or false, without having to use complicated if-else statements.
In this article, we will explore how to use AND and OR operators in Django Templates, as well as some best practices and advanced techniques to make your template coding more efficient and effective.
But first,
What are AND, OR operators in Django templates?
AND and OR are logical operators used to combine multiple conditions and evaluate whether a statement is true or false in a Django template.
When working with Django Templates, there are times when you need to combine multiple conditions to determine if a statement is true or false. This is where the logical operators AND and OR come in.
AND, OR operators can be used to create complex conditional statements that make it easier to write and read templates.
Let’s look, in detail, how each logical operator works in Django templates.
What is AND operator in Django templates?
In Django templates, the AND operator is a logical operator used to combine multiple conditions and evaluate whether all statements are true. The AND operator returns true only if all conditions are true. If any one of the conditions is false, the entire statement evaluates to false.
For example, you can use the AND operator to check whether two conditions are true.
If both conditions are true, the statement will evaluate as true.
If one or both of the conditions are false, the statement will evaluate as false. Using the AND operator in Django templates can help simplify your code and make it easier to read and understand, especially when you need to evaluate multiple conditions simultaneously.
What is OR operator in Django templates?
The OR operator is a logical operator used to combine multiple conditions and evaluate whether a statement is true or false in Django templates. The OR operator returns true if at least one of the conditions is true. If all of the conditions are false, then the statement evaluates to false.
Importance of AND, OR operators in Django templates
Using AND and OR logical operators in your Django template code can:
- Help simplify your code and make it easier to read and understand. Thus, allowing you to write more concise and readable code.
- Help you avoid writing too many multiple if statements for each condition you want to evaluate in your Django template code.
- Help you evaluate multiple conditions at the same time.
- Improve code efficiency by avoiding unnecessary conditional checking for conditions that may be already evaluated.
- Help you write complex logical conditions that can handle a wide range of use cases for your application.
AND operator in Django templates
Syntax of using the AND operator in Django templates
The syntax for using the AND operator in Django templates is fairly straightforward.
You can use the {% if %} template tag along with the AND operator to combine multiple conditions in a single statement.
Here is an example of the syntax for using the AND operator in Django templates:
{% if condition1 and condition2 %}
<p>Both conditions are true!</p>
{% endif %}
In this example, condition1
and condition2
are the two conditions that are being evaluated using the AND operator.
If both conditions are true, the contents of the if statement will be executed (in this case, a simple paragraph of text).
If either of the conditions is false, the contents of the if statement will be skipped.
Example code using the AND operator in Django templates
1. Example #1: Django e-commerce use case
Suppose you are building a Django web application that allows users to search for products based on various criteria, such as price range and product type.
You want to display only the products that match all the criteria specified by the user.
To accomplish this, you can use the AND operator in your Django template code to accomplish that.
Here’s an example:
{% for product in products %}
{% if product.price >= min_price and product.price <= max_price and product.product_type == selected_product_type %}
<div class="product">
<h2>{{ product.name }}</h2>
<p>Price: ${{ product.price }}</p>
<p>Product type: {{ product.product_type }}</p>
</div>
{% endif %}
{% endfor %}
In this example, products
is a list of product objects that are being looped over using a for loop.
Inside the loop, an if statement is used to check if the current product matches all the criteria specified by the user.
The AND operator is used to combine three conditions:
- The product’s price must be greater than or equal to the minimum price specified by the user,
- The product’s price must be less than or equal to the maximum price specified by the user, and
- The product type must match the selected product type.
If all three conditions are true, the contents of the if statement will be executed, which in this case is a div element containing the product name, price, and type.
If any one of the conditions is false, the contents of the if statement will be skipped for that product.
This allows you to display only the products that match all the user’s criteria, making it easier for them to find what they’re looking for.
2. Example #2: Blog use case
Suppose you have a blog that contains articles on various topics.
Each article has a category and a published date.
You want to display only the articles that belong to a specific category and have been published in the last month.
To accomplish this, you can use the AND operator in your Django template code. Here’s an example:
{% for article in articles %}
{% if article.category == selected_category and article.published_date >= cutoff_date %}
<div class="article">
<h2>{{ article.title }}</h2>
<p>Category: {{ article.category }}</p>
<p>Published on: {{ article.published_date }}</p>
</div>
{% endif %}
{% endfor %}
In this example,
articles
is a list of article objects that are being looped over using a for loop.Inside the loop, an if statement is used to check if the current article belongs to the selected category and has been published in the last month.
The AND operator is used to combine two conditions: the article’s category must match the selected category, and the article’s published date must be greater than or equal to the cutoff date (which represents one month ago).
If both conditions are true, the contents of the if statement will be executed, which in this case is a div element containing the article title, category, and publication date.
If either of the conditions is false, the contents of the if statement will be skipped for that article.
This allows you to display only the articles that meet both criteria, making it easier for readers to find articles that are relevant to their interests.
Best practices for using AND operator in Django templates
- Use parentheses to group conditions: When combining multiple conditions with AND, it’s a good practice to group them using parentheses to avoid any confusion in operator precedence. For example, instead of writing
if x == 1 and y == 2 or z == 3
, you can writeif (x == 1 and y == 2) or z == 3
. - Keep your conditions simple: Try to keep individual conditions as simple as possible to make it easier to understand what the code is doing. If you have complex conditions, consider breaking them down into smaller, simpler conditions.
- Use descriptive variable names: When writing complex conditions, it’s helpful to use descriptive variable names that make it easy to understand what the condition is checking. For example, instead of
if x > 5 and y < 10
, you can writeif number_of_widgets > 5 and total_cost < 10
.
OR condition in Django templates
Syntax of using the OR operator in Django templates
The syntax for using the OR operator in Django templates is fairly straightforward.
You can use the {% if %} template tag along with the OR operator to combine multiple conditions in a single statement.
Here is an example of the syntax for using the OR operator in Django templates:
{% if condition1 or condition2 %}
<p>Any of the conditions are true!</p>
{% endif %}
In this example, condition1
and condition2
are the two conditions that are being evaluated using the OR operator.
If any of the conditions are true, the contents of the if statement will be executed (in this case, a simple paragraph of text).
If either of the conditions is false, the contents of the if statement will be skipped.
Example code using the OR operator in Django templates
1. Example #1: “Love is in the air” use case
Suppose you have a website that sells products and you want to display a banner for a sale on either Valentine’s Day or White Day.
You can use the OR operator in your Django template to display the banner for either holiday.
Here’s an example code using the OR operator in Django templates:
{% if holiday == "valentine" or holiday == "white-day" %}
<div class="banner">
<h2>Save 20% on our special collection!</h2>
<p>Use code LOVE20 at checkout.</p>
</div>
{% endif %}
In this example,
holiday
is a variable that represents the current holiday being celebrated.If the value of
holiday
is either “valentine” or “white-day”, the banner code will be displayed.The OR operator is used to combine two conditions: either the holiday must be “valentine” or it must be “white day”.
If either of the conditions is true, the contents of the if statement will be executed, which in this case is a div element containing the banner text.
If both conditions are false, the contents of the if statement will be skipped, and no banner will be displayed.
This allows you to display the banner for specific holidays and encourage customers to make a purchase during the sale.
2. Example #2: Pet Lover Ecommerce use case
Suppose you have a website for a pet store and you want to display a message to customers who are either cat owners or dog owners.
You can use the OR operator in your Django template to display the message to either group.
Here’s an example code using the OR operator in Django templates:
{% if pet == "cat" or pet == "dog" %}
<p>Welcome, pet lover!</p>
{% endif %}
In this example,
pet
is a variable that represents the type of pet the customer owns.If the value of
pet
is either “cat” or “dog”, the message “Welcome, pet lover!” will be displayed.The OR operator is used to combine the two conditions: either the pet must be a cat or it must be a dog.
If either of the conditions is true, the contents of the if statement will be executed, which in this case is a simple message welcoming the customer.
If both conditions are false, the contents of the if statement will be skipped, and no message will be displayed.
Best practices for using OR operator in Django templates
1. Use parentheses to group multiple OR conditions:
{% if pet == "cat" or (pet == "dog" and age > 5) %}
<p>Welcome, pet lover!</p>
{% endif %}
If you have multiple conditions combined with OR, it’s a good practice to group them using parentheses. This can help ensure that the operator precedence is correct and that your code behaves as expected.
2. Use descriptive variable names:
When writing complex conditions, it’s helpful to use descriptive variable names that make it easy to understand what the condition is checking. For example, instead of, if x > 5 or y < 10
, you can write if number_of_widgets > 5 or total_cost < 10
.
How to use AND and OR operators together in Django templates
You can use the AND and OR operators together in Django templates to create more complex conditional statements.
These complex statements can be used to evaluate specific conditions and determine what content to display on our website. Just make sure to use parentheses to group the conditions together and ensure the correct operator precedence.
Syntax of using combined AND and OR operators
The syntax for using combined AND and OR operators in Django templates is straightforward.
You can group your conditions using parentheses to group more conditions and ensure the correct operator precedence.
When using both the AND and OR operators in Django templates, it’s crucial to use parentheses to group your conditions explicitly and ensure the correct order of evaluation.
Without parentheses, you might end up with unexpected results.
Here’s an example that demonstrates the importance of grouping your conditions correctly:
{% if pet == "cat" and age > 5 or pet == "dog" %}
<p>Welcome, pet lover!</p>
{% else %}
<p>Sorry, no pets allowed.</p>
{% endif %}
In this example, we are checking if the pet is a cat and if the age is greater than 5, OR if the pet is a dog.
Since the AND operator has higher precedence than the OR operator, the expression is evaluated as
(pet == "cat" and age > 5) or pet == "dog"
.So, the condition will be true if the pet is a cat and the age is greater than 5, or if the pet is a dog, regardless of the age.
To ensure that the conditions are evaluated as intended, we can group them using parentheses:
{% if (pet == "cat" and age > 5) or pet == "dog" %}
<p>Welcome, pet lover!</p>
{% else %}
<p>Sorry, no pets allowed.</p>
{% endif %}
By grouping the conditions, we can make sure that the evaluation order is correct and the condition is true only if the pet is a cat and the age is greater than 5, or if the pet is a dog.
Examples of using both AND and OR operators in Django templates
Here’s an example:
{% if (pet == "cat" and age > 5) or (pet == "dog" and age <= 5 and weight >= 10) %}
<p>Welcome, pet lover!</p>
{% else %}
<p>Sorry, no pets allowed.</p>
{% endif %}
In this example, we are using parentheses to group the conditions and ensure that the AND operator is evaluated before the OR operator.
We are checking if the pet is a cat and if the age is greater than 5, OR if the pet is a dog and if the age is less than or equal to 5 and if the weight is greater than or equal to 10.
Best practices for using AND and OR operators together
When using the AND and OR operators in Django templates, it is important to keep in mind their order of operations.
The AND operator takes precedence over the OR operator, which means that AND conditions are evaluated first, followed by the OR conditions.
It’s also worth noting that you can use parentheses to group conditions and force a certain order of evaluation.
How to use NOT operator in conjunction with AND and OR operators
In Django templates, you can use the not
operator to negate a value or expression. You can use the not
operator in conjunction with the and
and or
operators to create more complex conditions, that can be negated.
You can also use parentheses to group expressions and control the order of evaluation.
For example:
{% if not (condition1 and condition2) %}
<!-- code to be executed if either condition1 is false or condition2 is false -->
{% endif %}
In this example, the and
operator is evaluated first, and then the not
operator is applied to the result, meaning it will be the complete opposite of the evaluation.
Example use of NOT operator with AND and OR operators
Negation is commonly used in programming when we want to check if a condition is not true.
Let’s say we have a list of items that we want to display on a webpage.
We want to show a message to the user if the list is empty, but not show anything if the list is not empty.
We can use the not
operator to check if the list is empty, like this:
{% if not items %}
<p>There are no items to display.</p>
{% endif %}
In this example, the if
statement checks if the items
list is empty. If the list is empty, the condition evaluates to, True and the message “There are no items to display.” will be displayed on the page.
If the item
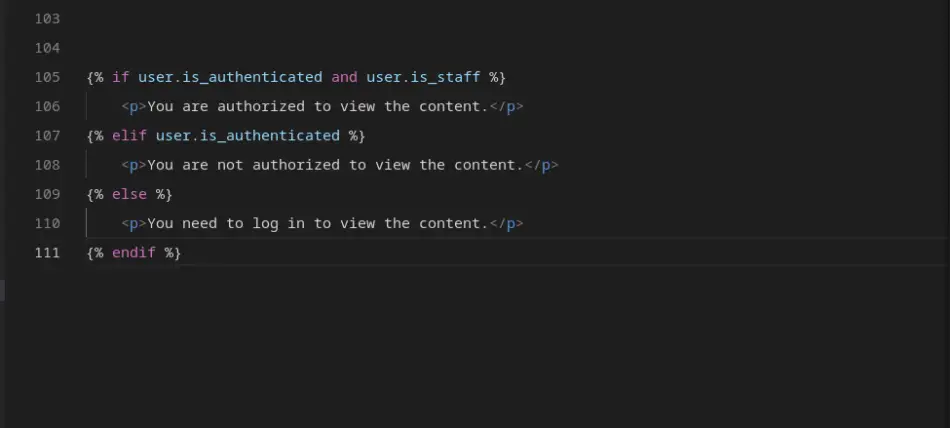
s
list is not empty, the condition evaluates to, False
, and the message will not be displayed.
This is a simple example, but negation can be used in many more complex scenarios in Django templates.
For example, we can use negation to check
- if a user is not logged in,
- if a form field is not valid, or
- if a database query returned no results.