Django block Tag: How to use {% block content %} in Django
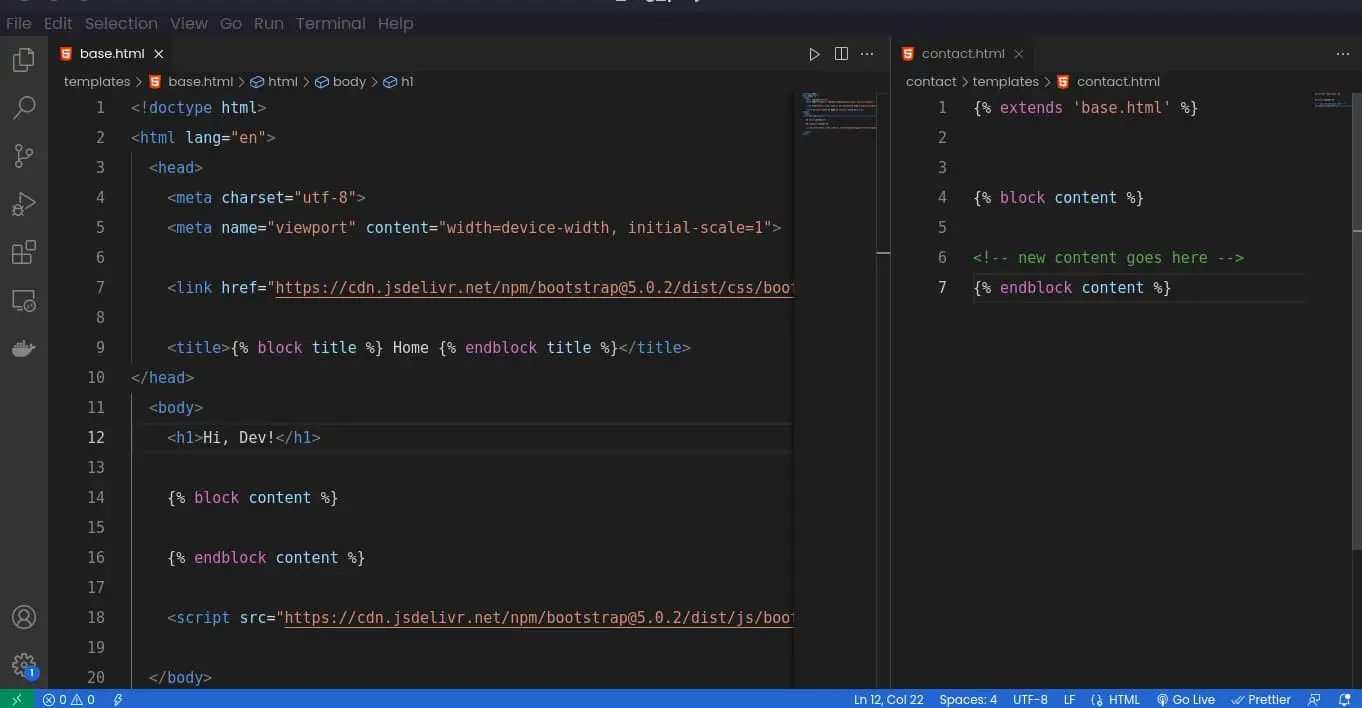
When using Django for web development, Django provides web developers the ability to achieve the DRY (Don’t Repeat Yourself) principle with ease. And when it comes to reusing certain parts of a .html file or whole HTML templates, Django provides the block tag that can be overridden by a child template and template inheritance to achieve that.
What is {% block content %} in Django?
The {% block %} tag acts as a placeholder where the content that you place in this block can be replaced by the content that you define in the child template using the same block tag name.
The {% block content %} tag used in Django templates allows you to define a replaceable block of code that can be used as default or be overridden by a child template to add unique data specific to that template.
This means that you can create a section in a parent template (base template) that has unique styling. Whenever you use this block in your child template (e.g contact.html) the section styling can be rendered without having to repeat the styling rules used in the parent template.
The other thing is that you can use the same name of the block in your child templates and add unique data that overrides the content declared in the parent template.
What is the use of block content in Django?
{% block content %} can be useful when:
- You want to have a uniquely-styled section in your templates that can be overridden without having to rewrite HTML and CSS code again.
- Achieving template inheritance where the {% block content %} tag is used to define blocks of content in the base template that can be overridden by the child templates.
- {% block content %} helps achieve modular design across your website. The structure of a website can be reused while still allowing for the customization of specific parts of a web page.
- Using block content can save time in the development process because it makes it possible to reuse the structure and styling for specific parts of a web page.
What is the difference between block content and Django template inheritance?
When using the Django Template Language, Django provides two important features for creating reusable templates or parts of a template: {% block %} template tag and template inheritance. While both play a crucial role to achieve a modular website design, they serve distinct purposes.
Here’s a comparison table to help understand the differences between {% block content %} tag and template inheritance:
{% block content %} | Template inheritance |
---|---|
Declares blocks of content in a template file (parent template) that can be overridden by other templates called child templates. | Template inheritance enables the creation of reusable templates by creating a parent template that has styling and data that is similar across the templates that inherit from it. |
Helps create a placeholder for content that can be customized in child templates. | Provides an approach to reuse the structure of a base template while still allowing for customization and the addition of specific parts in a child template. |
{% block %} tags can be used multiple times in a template | Extending a parent template can only be achieved using a single line of code in a child template. |
What is {% endblock content %} used for in Django?
The {% endblock %} tag in Django is used to declare the end of a block defined using the {% block %} tag. Therefore, whenever you declare a {% block %}
tag in Django templates, you must end the block with the {% endblock %}
tag.
Most programmers will use {% block content %} to declare a block in Django templates.
To make it easier to identify the end of a block, you can always use the name of the block in your {% endblock %} if your code gets larger. {% block content %}
is used to end a block called ‘content’.
Why are block tags important in Django templates?
By using {% block %} tags in Django, you allow yourself to:
- Achieve code reusability and reduce duplicated code in your Django templates.
- Achieve customization by overriding certain parts of your web pages.
- Create modularized web application design where templates are broken down into sections that can be managed easily.
- Achieve readability of your code when you organize specific code blocks into manageable and identifiable parts.
Example project using block tag in Django
Let’s take a closer look into how you can use {% block content %} tag in your base and child templates. By creating a base template, I will demonstrate how block tags can be used to create reusable templates with customizable content.
Whether you’re a seasoned Django web developer or just starting out, this example project will give you a deeper understanding of {% block %} tags and their importance in the Django template system.
Here are the steps you need to take to create an effective {% block %} tag in Django:
- Create a Django project and app
- Configure templates directory
- Create a base template
- Create a {% block content %} tag in the base template
- Override {% block content %} in child templates
Let’s see how each step can be achieved in a real-world project.
Create a new Django project and app
First, let’s create a new Django project for demonstrating how to use {% block content %} in Django templates.
Here are the actionable steps to create a new Django project:
Open the terminal and create a new Django project folder if you don’t have one
# navigate into the Desktop folder or your desired location
cd ~/Desktop
# Create a new directory
mkdir block_tag_project
# navigate into the new directory
cd block_tag_project
Create a new virtual environment for the project
Execute the following commands to create a new Python virtual environment:
python3 -m venv block_tag_project_env
Activate the new virtual environment
Run the following command to activate your newly created virtual environment.
source block_tag_project_env/bin/activate
Install Django
pip install django
After installing Django, create a new Django project and app using the following commands:
# Create an new Django project
django-admin startproject btp .
# Create a new Django app
./manage.py startapp contact
Open the project workspace with your favorite code editor or IDE and add contact
app to the list of installed apps in your Django project settings.
Open the project folder using your code editor
code .
Add contact
to the list of installed apps.
In your settings.py file:
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
# add you app here
'contact',
]
How to configure templates directory
After creating a Django project and creating a new app, the next step is to configure the templates directory that we’ll use to store a base template.
Here are the actionable steps to configure the templates directory in Django:
1. Create a new directory called templates
in your project root directory
Open the terminal and navigate into the project root directory. Then, type the following command:
mkdir templates
2. Open settings.py
file and scroll to the TEMPLATES
section
Replace the section with the following code:
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
# new line of code to configure Django to use project-level templates
'DIRS': [BASE_DIR / 'templates'],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
3. Save the settings.py
file
You have now completed configuring Django to use the project-level templates directory that we will use to create the parent template.
How to create a base template (base.html) in Django
After configuring the templates directory, follow these steps to create the base template for the example project:
I. Open the Terminal and navigate into the templates directory
# use cd command to change directory
cd templates
II. Create a new file (base.html) inside the project-level templates directory
touch base.html
With the new base template file (base.html), you can write HTML and CSS code that you wish to be consistent across your website.
Here’s a starter .html template code for the base template with Bootstrap CSS framework loaded.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Hello, world!</title>
</head>
<body>
<h1>Hi, Dev!</h1>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
</body>
</html>
How to create {% block content %} in Django base template
With the new template, we have achieved template inheritance. Let’s add some block tags to achieve customizable parts of the base template.
Open base.html
file using your favorite code editor.
code templates/base.html
Inside, the <body> tag, create a new block using the following syntax:
{% block content %}
{% endblock content %}
With the following configuration, you can be able to create a block that can be overridden across your website.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Hello, world!</title>
</head>
<body>
<h1>Hi, Dev!</h1>
{% block content %}
{% endblock content %}
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
</body>
</html>
Override {% block content %} in child templates
In your child template, you can be able to override the `{% block content %} tag by creating the same block with the same name in your child template.
Follow these steps:
Create a new template, contact.html for your contact app
# create a templates directory for the contact app
mkdir contact/templates
# Create a new .html file
touch contact/templates/contact.html
Open the contact app, contact.html
file and follow these steps to override the {% block content %} block:
Step I: Add {% extends 'base.html' %}
at the top of the child template
In contact/templates/contact.html
:
{% extends 'base.html' %}
By adding {% extends … %} in your template, Django can be able to recognize the .html file as a child template and inherit the styling and any {% block %} tags.
Why is {% extends %} tag used in Django templates
The {% extends %}
tag is used to achieve template inheritance where a .html template (child template) can inherit from another template (parent template). The tag specifies the parent template the child template will extend from.
Step II: Override {% block content %} by declaring it in your child template
In contact/templates/contact.html
:
{% extends 'base.html' %}
{% block content %}
<!-- new content goes here -->
{% endblock content %}
By using the same name of the block used in the parent template, a child template can override the contents of the parent template with new content.
That’s how you use {% block %} tags to achieve template inheritance for specific sections or your Django templates.
Other sections that can be implemented using {% block %} tags are:
- Your <head> section , {% block title %}
- Customized CSS in each child template, {% block css %}
- Customized JS code in each child template, {% block js %}
Best practices for using block content in Django templates
Here are some best practices for using {% block %} tags in Django templates:
- Consistent naming conventions: Use consistent naming conventions for your blocks. For example, you can use snake_case to name your block if they require more than one word.
- Provide meaningful names for your blocks: Choose meaningful and descriptive names for your template blocks.
- Avoid overusing blocks: Avoid using blocks for every small piece of code, as it can lead to many small and difficult-to-maintain blocks.
- Avoid over-nesting in your blocks: When you have nested blocks, use shallow nesting to avoid complex template files.
How to troubleshoot Django block content not showing
Here are actionable steps to troubleshoot Django {% block content %} not showing:
- Make sure that {% block content %} is properly defined in the parent and child templates.
- Verify that the {% block content %} tag name of your child template matches exactly that of the parent template, including the use of case-sensitive characters.
- Make sure you have the correct template configuration in your
settings.py
file. - Ensure you are referencing the correct parent filename and its correct location.
- Check for typos and syntax errors and ensure you have closed your {% block content %} tag with
{% endblock %}
tag.
How to use block title in Django
If you wish to have unique page titles across your website, you can create a customizable {% block title %} tag in your templates.
To use a block named “title” in Django templates, you will need to define it in a parent template and then override it in a child template.
Here are the steps to take to use {% block title %} in your Django templates:
1. Define the block in your parent template
In the parent template, add a block named “title” using the following syntax: {% block title %} Home {% endblock title %}
.
<title>{% block title %} Home {% endblock title %}</title>
2. Override the block in your child template
In the child template, extend the parent template and override the block named “title” using the following syntax:
{% block title %} Contact page {% endblock title %}
Can you extend two HTML templates in Django?
You cannot inherit two HTML templates in Django templates by declaring more than one {% extends %} tags. The Django template inheritance system allows for a single template to be extended by a child template.
Thus, you can only inherit a one parent template in a child template.
Such informative content. Thanks, Stephen