What are Views and URLs in Django: How to create a view and URL
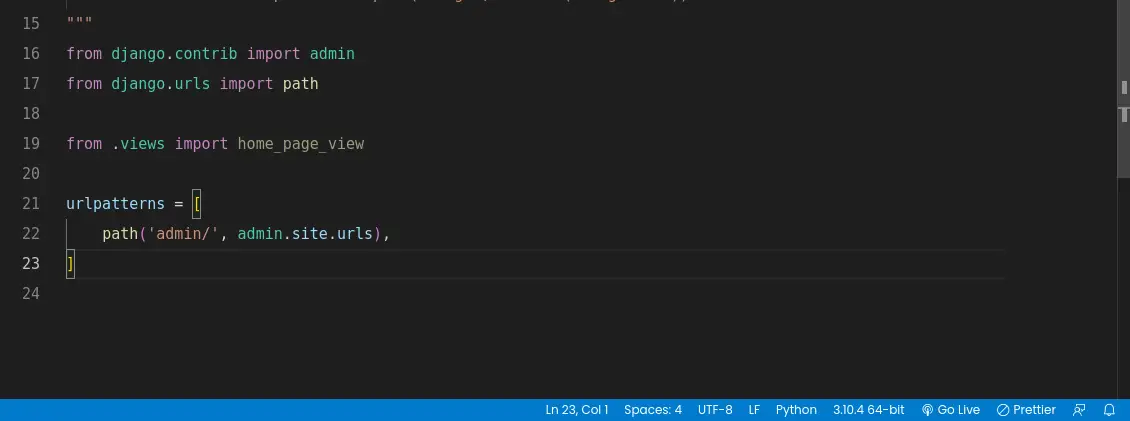
The views and URLs form your website’s core functionality when developing a Django web application.
Without the views and the URLs, your Django web application is non-functional – it misses the core function of server-client communication.
Django Views and URLs are used to create web pages and links for your website. Views are used in creating web pages, while URLs are used to create links that will be available for your website users to access.
Without the views and URLs in Django, your web application cannot handle requests, process them, and return a response to the user upon typing the domain name on their browser.
To understand Django’s views and URLs, you must first know how the web works.
How the web works
The web works in a way that we have two parts of an interaction: the client application as one part and the other part as the server.
A client application, for example, is a web browser such as Chrome, which a visitor of your website uses to access the files of your Django web application.
The user enters the address of the location of your web application files to trigger a request. This address is called an IP address. An IP address is usually in the form of numbers, for example, 1.89.95.67, representing the location of your website’s files.
Now, that number would be very hard to remember every time you want to visit a particular website.
The best way to remember the IP address is to associate it with a name you can easily remember, such as mypetwebsite.com.
Domain name resolving helps translate IP addresses into domain names that are easily remembered.
So, after a user visits the home page of our website by typing mypetwebsite.com, the client application triggers a request to the server part of your web application.
The client requests the server to provide the contents of our website’s home page.
How does the server respond?
The server part of your website is the hosting machine of your website.
Django website files, web server, and the database live on the server-side.
Here are the best web hosting services you can purchase for your Django applications, WordPress, or any other website: Most Trusted Web Hosting Services.
The web server is responsible for managing website files and requests made to your Django application.
Upon receiving the request, the web server routes the request to the Django application for processing.
Django matches the URL endpoint, which in our example, is an empty string set for the home/index page and maps it to the appropriate view.
The view takes the request, processes it, and returns the result using the same URL endpoint.
The web server takes the response from your Django application and routes it to the client that requested it.
In this case, the data returned by the view will be an HTML page of our Django application.
The same request-response strategy happens when users constantly interact with your Django website.
Make a request by visiting a contact page as the URL and the view associated with that URL returns a response containing your contact page’s contents.
How Django web pages and URLs work
Views and URLs interact together to create the functionality of web pages and the URL endpoint of a Django application.
URL endpoints are the links, for example, mypetwebsite.com/contact, that visitor of a website use to make request to a web server.
Upon making that request, Django matches the URL endpoint with the suitable view that processes the request and returns the response to your website visitors.
If you have a view function in your views.py, connect it to a URL inside the urls.py to allow request routing.
When a client requests a page by accessing a URL, Django matches the URL pattern by iterating through the list of all the URLs in your project URL configuration.
If Django matches that particular URL pattern, Django uses the view connected to the URL endpoint.
The view processes the information and returns an HTTP object to the user. Mainly, the HTTP object includes an HTML page of the URL endpoint requested.
If you use Django to create APIs, each view returns text data rendered on your web application’s frontend.
The frontend side, which may be implemented using frontend frameworks such as React, is responsible for generating the HTML page and using the data from the API.
Whether a page is generated by Django or a frontend framework, Django views and URLs operate together to handle requests and provide responses made to your Django web application by client applications.
Let’s create a simple Django web application to illustrate how views and URLs interact to achieve web page functionality for your Django application.
Implementing URL and View functionality in a Django Project
Create a new Django project. Check this article that illustrates how to install Python, create a new virtual environment, and install Django.
Activate the virtual environment and create a new Django project.
django-admin startproject djangoWeb .
Open the project folder using your favorite IDE or code editor.
Create a new file with the name views.py inside the main project folder. Create the file in the same folder that has your settings.py file.
touch djangoWeb/views.py
After creating the Django project, how do you create a view in Django?
You create a view in Django by using a Python class or function.
Views created using Python functions are called function-based views. They are created in the same way functions are declared in Python.
To create a function-based view, define the function name and pass the request object as the arguments.
The request object will be responsible for taking the request made by the user using the GET method, for example, and then passing the request to the view for processing.
After creating the views.py file, write a new function-based view that will return a simple message to the user upon requesting the/contact/ URL endpoint of our Django project.
from django.http.response import HttpResponse
def contact_page_view(request):
return HttpResponse('You are viewing the contact page')
Here, we’re importing the HttpReponse function, which the view will use to return a simple HTTP object containing text data.
Next, the new function contact_page_view() uses the HttpResponse to return a simple text to the user upon accessing the contact page of our web application.
Open the main project urls.py file, import the contact_page_view() function, and connect the view to the /contact/ URL endpoint.
Here’s an example code for connecting views and URLs for your Django application.
from django.contrib import admin
from django.urls import path
# import the view
from .views import contact_page_view
urlpatterns = [
path('admin/', admin.site.urls),
# connect the view to the /contact/ URL endpoint
path('contact/', contact_page_view),
]
Run the default Django development server, python manage.py runserver, and open 127.0.0.1:8000/contact
You should see a similar page.
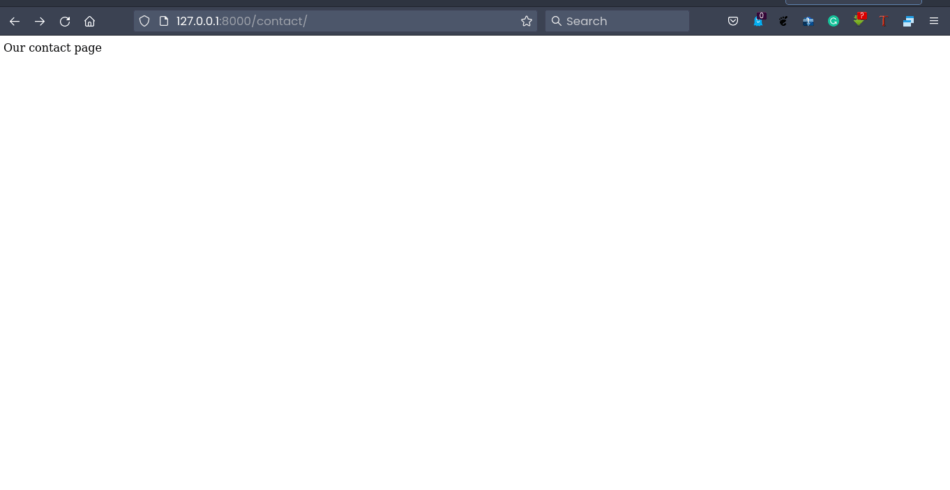
See how easy it is to connect Django views and URLs.
To summarize how views and URLs work, upon accessing 127.0.0.1:8000/contact, I am requesting the Django development server to connect to the appropriate view and display the page’s contents.
Django matches the URL endpoint /contact/ and uses the connected view, contact_page_view, to process the request.
The contact_page_view receives the request, processes it, and returns the simple text message to the user.
Views create web pages, and URLs define the links available in your Django web application.
That’s it basically for this article.
I hope that you have understood the basics of Django views and URLs.
If you have any questions, drop them in the comment section.