How to fix “No module named ‘psycopg2′” in Python Django?
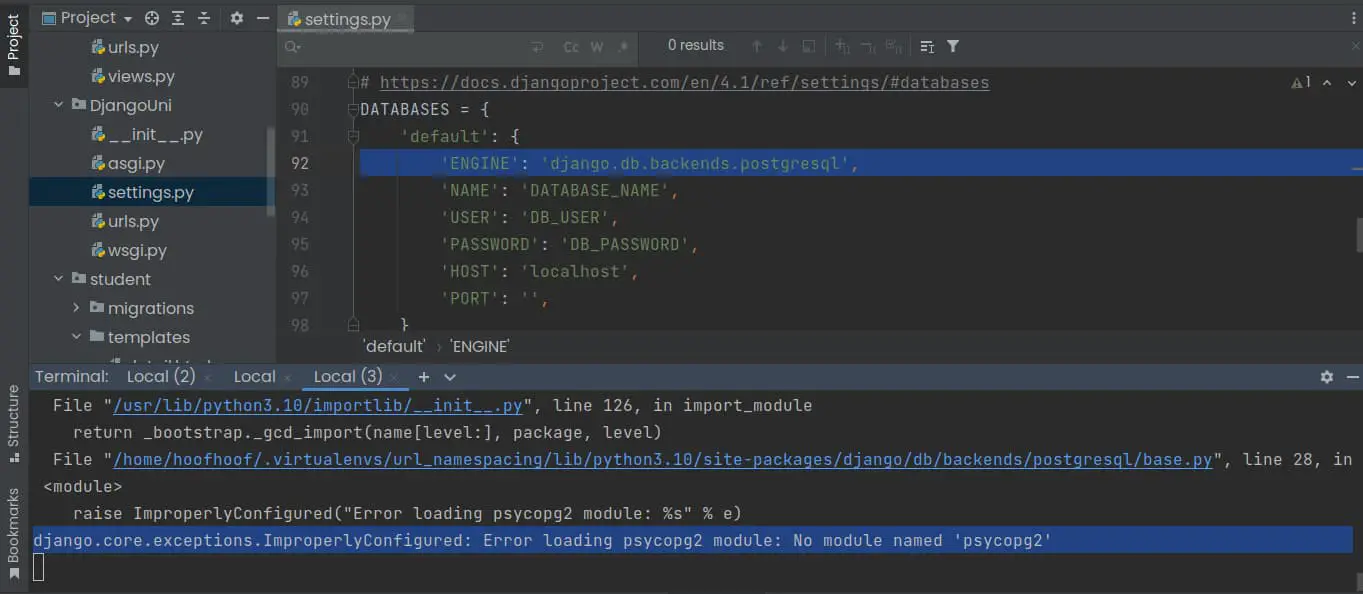
Django is a powerful and feature-rich framework that allows you to build web applications with database capabilities. However, when working with Django and database management systems such as PostgreSQL, these two technologies are different as they are made using different languages.
Thus, you must have an intermediary that allows the two technologies to communicate and interact. An example of an intermediary is the psycopg2 database driver that enables you to perform database operations on PostgreSQL using Python.
But even with the best tool, you can sometimes have some frustrating moments when dealing with database connections. Whether you have a misconfigured DATABASE setting, syntax error, or mistyped code, these errors can be pretty disappointing to troubleshoot and fix.
One common exception you may get when working with PostgreSQL and Django is the ModuleNotFound Exception. The exception is just a simple way that Django tells you that it cannot find the file referenced in the import statement.
Thus, the psycopg2 binary cannot be located because either it has not been installed in your Python virtual environment or you have a misconfigured setting.
Let’s look in detail at the reasons that would lead you down the path of connection errors with the psycopg2 module.
Reasons why psycopg2 is not working with Django
There are a couple of reasons why the psycopg2 module may not be working in your Django project:
- The psycopg2 binary is not installed in your virtual environment.
- For Python versions < 3.4, you have not installed psycopg2-binary binary in your Python virtual environment.
- You have not configured Django to use the psycopg2 module in your database ENGINE setting.
- You have a misconfigured database connection that could arise from incorrect or mistyped database engine settings.
Here is a detailed explanation as to why you may have database connection details when working with PostgreSQL and Django and the approach to fix the error.
The psycopg2 module is not installed in your Python virtual environment
When working with Python Django and PostgreSQL databases, a database driver is needed for the two technologies to communicate. Thus, you must install the psycopg2 database driver in your Python virtual environment you have installed Django.
The most common contributor to the exception “django.core.exceptions.ImproperlyConfigured: Error loading psycopg2 module: No module named ‘psycopg2’” in Django arises from not installing the psycopg2 module.
Thus, to fix the ‘No module named psycopg2’ error, you must install psycopg2 in your virtual environment.
Here are the steps to fix the No module named ‘psycopg2‘ in Django by installing the psycopg2 module.
Step 1: Activate the virtual environment you have installed Django
Open the Terminal window and type the following
source path_to_env/bin/activate
# example
source ~/.virtualenvs/DjangoBlogEnv/bin/activate
Step 2: Install the psycopg2 module using pip
After activating the virtual environment, type the following command to install the psycopg2 module.
pip install psycopg2
Step 3: Add ‘django.db.backends.postgresql’ as the default ENGINE
After installing psycopg2, you must configure Django to use PostgreSQL as the default database.
Open the settings.py file and locate the DATABASES dictionary.
Add ‘ENGINE’: ‘django.db.backends.postgresql’, line.
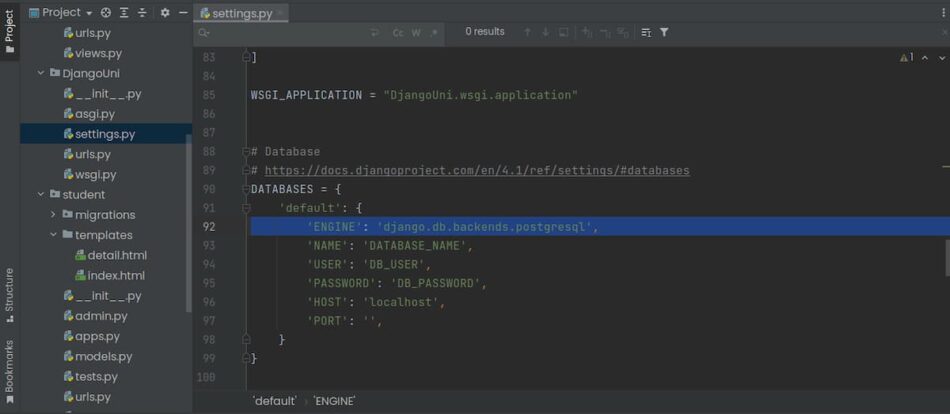
Step 4: Run your migrations
You should run your migrations now that you have installed psycopg2 and configured Django to use the PostgreSQL database engine.
python manage.py migrate
Try rerunning your Django development server; you should not get the ModuleNotFound exception again.
python manage.py runserver
The psycopg2-binary module is not installed for Python versions lower than 3.4
If you use a Python version lower than 3.4, installing psycopg2 will not work for your Django project due to concurrency problems with binary packages. Instead, you should install psycopg2-binary to use PostgreSQL with Python.
Here are the steps to configure the Django project using a Python version lower than 3.4 to use PostgreSQL.
Step 1: Activate the virtual environment you have installed Django
Open the Terminal window and type the following
source path_to_env/bin/activate
# example
source DjangoBlogEnv/bin/activate
Step 2: Install the psycopg2-binary module using pip
After activating the virtual environment, type the following command to install the psycopg2-binary module.
pip install psycopg2-binary
Step 3: Add ‘django.db.backends.postgresql’ as the default ENGINE
After installing psycopg2, you must configure Django to use PostgreSQL as the default database.
Open the settings.py file and locate the DATABASES dictionary.
Add ‘ENGINE’: ‘django.db.backends.postgresql’, line.
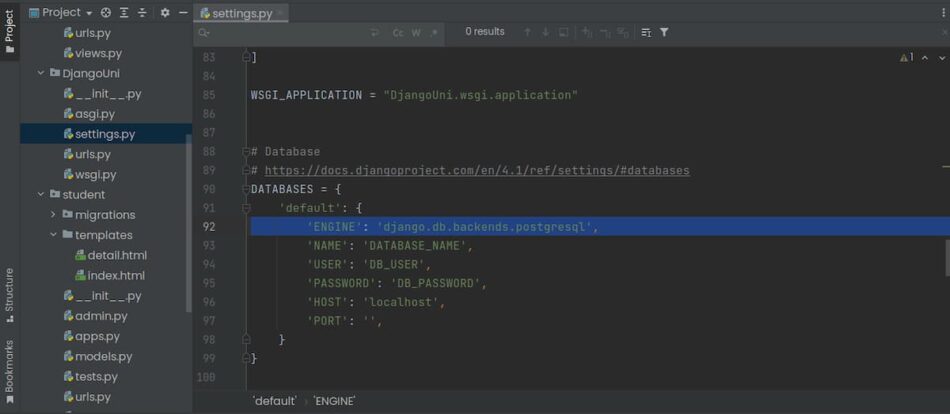
Step 4: Run your migrations
You should run your migrations now that you have installed psycopg2 and configured Django to use the PostgreSQL database engine.
python manage.py migrate
Try rerunning your Django development server; you should not get the ModuleNotFound exception again.
python manage.py runserver
Not configuring Django to use psycopg2 module in your database connection settings
If you have not configured Django to use the psycopg2 module, you will likely get an error when connecting Django and PostgreSQL. Another reason you may have a ‘ModuleNotFound: no module named psycopg2’ error in Django is that you have a mistyped database connection settings.
For example, having a mistyped ENGINE value will lead to a ModuleNotFound error.
Check that you have configured your Django DATABASES dictionary well.
Use the following code for reference:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'DATABASE_NAME',
'USER': 'DB_USER',
'PASSWORD': 'DB_PASSWORD',
'HOST': 'localhost or AWS RDS URL',
'PORT': '5432',
}
}
The default port for connecting the PostgreSQL database to Django is 5432. Thus, in your Django settings, do not forget to specify the PORT. Besides, the PORT value should be a string.
However, you are at liberty to use another port number provided other services on your Linux system do not use it. When using a different port for your PostgreSQL database, you need to specify that particular port number in the PORT field.
How to fix ModuleNotFound: no module named psycopg2
There are a few reasons why your PostgreSQL connection with Django is not working while using the psycopg2 module. To fix the ModuleNotFound: no module named psycopg2 in Django, check that you have the following.
- Installed psycopg2 or psycopg2-binary installed in your Python virtual environment. You can install the psycopg2 binary by activating your Django project virtual environment and executing
'pip install psycopg2'
or'pip install psycopg2-binary'
- Configured Django database connection details correctly. A misconfiguration in your DATABASES setting in your Django settings is a common cause for the ModuleNotFound exception. Ensure you have correctly typed the database ENGINE value. Check that you have the following line in your DATABASES settings variable:
'ENGINE': 'django.db.backends.postgresql',
- You have activated the correct virtual environment that has the psycopg2 installed. When using a virtual environment, make sure you have activated it by running
source path_to_env/bin/activate
command before your try to use psycopg2. - Installed all the dependencies, including the
postgresql-libs
, on your Linux machine.
Related Questions
How to install psycopg2 in VS Code?
When using Visual Studio Code, you can install psycopg2 easily by following these steps:
Step 1: Open VS code and activate the virtual environment for your Django project
Open the terminal window in visual studio code by pressing CTRL + `
Type the following command to activate the virtual environment:
source /path_to_your_env_folder/bin/activate
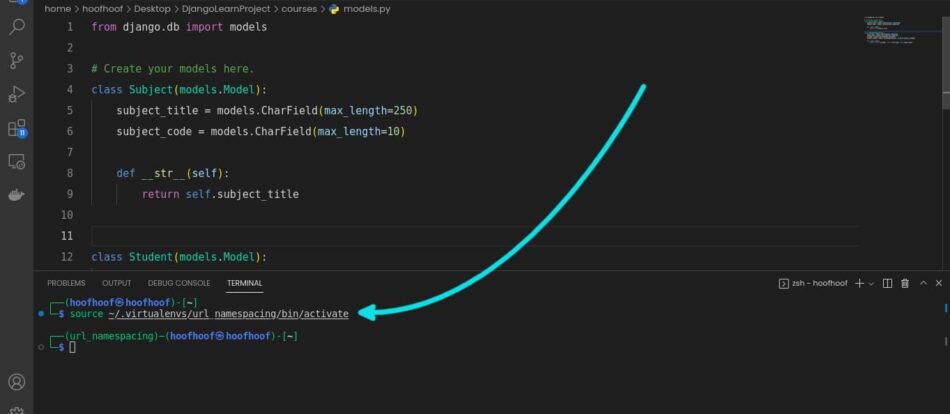
Step 2: Install psycopg2 using pip
After activating the virtual environment, type the following command to install psycopg2 or psycopg2-binary module using pip.
pip install psycopg2
# or
pip install psycopg2-binary
After you have successfully installed the psycopg2 module, you should be able to connect PostgreSQL to your Django project.
How to install psycopg2 with pipenv
Follow these steps to install psycopg2 using pipenv:
Step 1: Navigate into your project root directory
Using the Terminal, navigate into the root directory of your Django project using the cd
command.
cd /path_to_project_directory/
Step 2: Activate pipenv environment using pipenv shell
command
pipenv shell
Step 3: Install psycopg2 using pipenv
Install the psycopg2 module using pipenv by running the following command:
pipenv install psycopg2
If you have Python version 3.4 and lower, use the following command:
pipenv install psycopg2-binary
After a successful installation, you may configure your Django or Python project to use the PostgreSQL database.
How do I know if psycopg2 is installed?
Python provides several ways to check if psycopg2 is installed in your virtual environment. You can use either of the following ways to ensure you have installed psycopg2 correctly:
Check the list of installed modules using Python
Inside your Python project, create a new file called check.py and paste the following code:
from importlib import util
if util.find_spec("psycopg2"):
print("psycopg2 is installed")
else:
print("psycopg2 is not installed")
Activate the virtual environment you want to check if psycopg2 is installed.
source /path_to_env/bin/activate
Run the Python file by executing the following command:
python check.py
You should get a result indicating whether psycopg2 is installed.
Check your requirements.txt file or run pip freeze
to see whether psycopg2 is installed
If you have a requirements.txt file, you can check whether psycopg2 or psycopg2-binary is listed.
Besides, you can run pip freeze
command to check psycopg2 installation. You should see psycopg2 listed if it is installed.
Otherwise, you will not find it. Because of that, you have to install psycopg2 by running pip install psycopg2
Can psycopg2 connect to MySQL?
You cannot use psycopg2 as a database adapter for MySQL. Psycopg2 is a database driver designed for PostgreSQL database management system and is incompatible with MySQL.
If you wish to use MySQL database in your Django project, you need to install a different database driver such as mysql-client. The mysql-client adapter is designed to connect to the MySQL database when using Python.
To use the MySQL database with Django, you need to modify your Django project settings to interact with the MySQL backend.
First, install mysql-client by executing the following command:
pip install mysql-client
Secondly, open your project settings.py file, and inside the DATABASES variable, replace the ENGINE dictionary key to look like this:
DATABASES = {
'default': {
# replaced line
'ENGINE': 'django.db.backends.mysql',
'NAME': 'DB_NAME',
'USER': 'DB_USER',
'PASSWORD': 'DB_USER_PASSWORD',
'HOST':'localhost',
'PORT':'3306',
}
}