Difference between null=True and blank=True in Django?
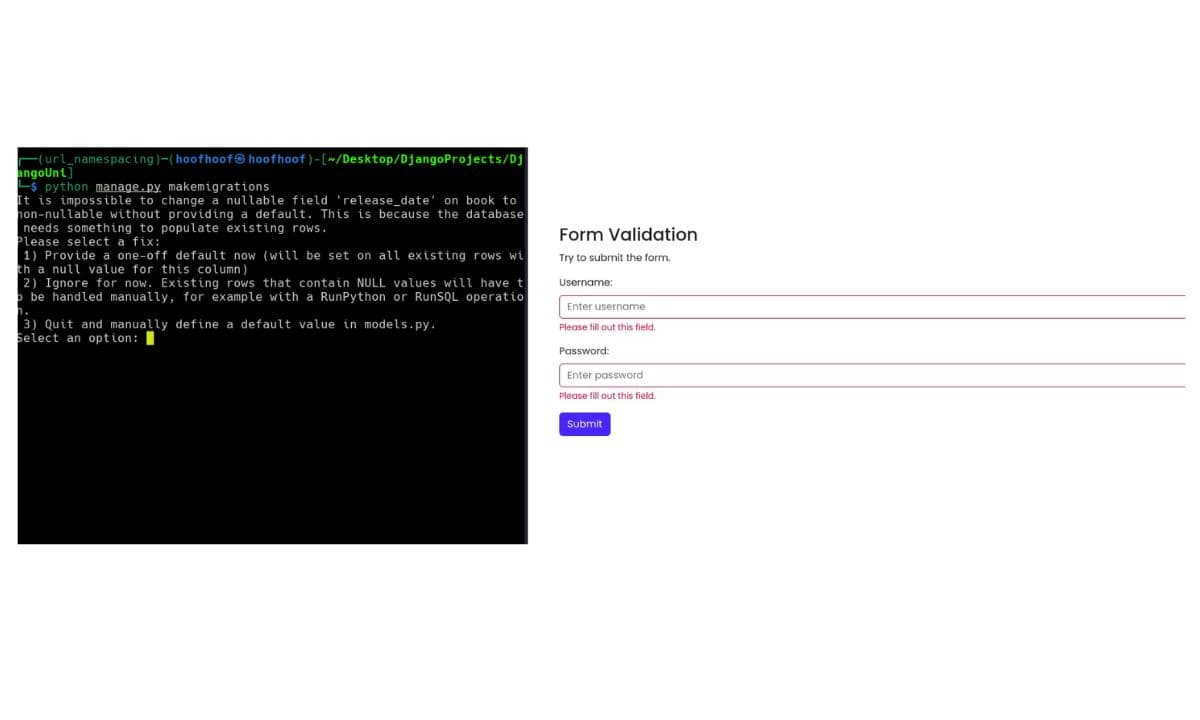
Django provides a couple of model field options, such as null
and blank
that allow you to customize the appearance or behavior of the field in your forms or admin site pages and data stored in the database. These model field options are defined as parameters in the Django model field if you wish to add them.
A model field in Django represent the structure of the database column defining the data types to store for a particular type of data. Each field is represented by a class attribute of the model class and maps to a specific data type such as strings, integers, booleans, dates, etc.
Therefore, through a Django model field, you can define whether a database table column should store integers, strings, e.t.c.
Example of the model field:
# a blog post model class BlogPost(models.Model): # example of a model field in Django title = models.CharField(max_length=100)
Within these Django model fields, you can define extra options such as default values; maximum characters used in that particular field; whether a database table column should have NULL values, default values, empty strings, etc.
Some examples of the model field options are the null
and blank
field attributes that specify whether a field can accept blank values in the form fields and the database.
Here are the differences between null
and blank
model field options:
null=True | blank=True |
---|---|
Specifying null=True argument in Django model fields means that the database will accept null values in the associated table column. | Specifying blank=True argument in Django model fields indicates that the form can accept empty entries for the associated form field. |
null=True validation happens at the database level. Therefore, null determines whether a database table column can have NULL or empty values. | blank=True validation occurs at the form level. blank determines whether a field will be required in the form of a frontend interface such as the admin site page or customized form. |
The database must accept a null value or an empty string, “”. | With blank=True , the database can accept a null value, an empty string, or an actual value. |
Example code for specifying null=True in Django model fields:model_field = models.DateField(null=True) | Example code for specifying blank=True in Django model fields:model_field = models.DateTimeField(blank=True) |
Let’s go into detail about how blank and null model field options affect data entry on the fronted interfaces and the database.
What happens when you specify the null field option in Django? (Setting null=True
)
Every time you provide a Django model field with a field option null
that is set to True, null=True
, Django allows the field to accept NULL values in the database. With such a configuration, the database column does not have any value whenever each model instance object is created or updated.
Thus, if you have a model field in Django like birth_date and you provide null=True
as one of the field options, Django will allow the database column birth_date to accept NULL values or rather an empty date value.
class Person(models.Model):
first_name = models.CharField(max_length=255)
last_name = models.CharField(max_length=255)
# The database may have a NULL / empty value for this field
birth_date = models.DateField(null=True)
With the Model example above, it is possible to create a Person instance object without providing the value for the birth date. Django will create the person object without requiring a value for the birth date column because null
is set to True
.
If you try to create an object, Django should not throw an exception.
new_person = Person.objects.create(first_name="Luke", last_name="SkyWalker")
If you use
objects.create
manager method, you should not specify the fields that are set to null,null=True
to None or an empty string.As they are not needed when creating the objects, you should not specify them in your create model manager method.
So, what is null=True in Django? Specifying null=True
in your Django model fields, you can control data validation at the database level by allowing the field to have a NULL or empty value. Thus, each database column of every object with the null=True field option will accept a NULL or empty value.
Depending on the database management system, a model field that accepts string values and has
null=True
can accept two possible values for no data: NULL or an empty string.
Effect of setting null=False
in Django
By default, null
model field option is set to False in Django. Upon model field definition, Django expects the field to have a value provided when creating or updating an object instance.
Thus, setting null=False
explicitly may not be necessary.
Because null
is set to False
by default, it means that the field is required to store a value, whether explicitly defined or not. Thus, it cannot store NULL values.
Examples of scenarios where null=False
is needed in Django:
- Accepting email fields. When using the email field for authentication and communication, it is a good practice to accept mandatory values because you will need the email the user provides.
What is blank=True in Django?
blank=True
in Django specifies that a Django model field should accept no value when tabulating data on a custom form or in the admin site pages.
Missing a value for the associated field on a form, Django will not throw a validation error on the form a user uses to enter data.
Note: Although the form field can be left empty, it is worth noting that Django may throw an exception if the value of the field lacks when creating or updating an object. This happens when you do not specify a default value or a custom validation during saving.
A solution to this problem is to:
- Add
null=True
in your model field so that Django may accept NULL or empty values in the database.- Perform extra validation by explicitly setting the value of the field (if not provided by the user). This can be done by overriding the save method to include extra validation that sets the value of the missing value. An example of this would be where you do not want a logged in user to enter their username. Instead, you automatically set the value of the username field in the save() method.
- Specifying a default value for the model field with
blank=True
option.
Effect of setting blank=False
in Django
By default, setting blank=False
on a field have no effect because Django already sets each field to accept values. Thus, each field created in Django will have blank=False set by default, indicating that the form field must have a value when creating or updating an object.
A good example of such a form validation is when you want a user to provide an email address in your site’s contact form. Well, having null set to False
(left to default) in the email model field will instruct Django to throw an error when a user does not provide a value in the email field.
Setting blank=False
on a field or leaving it to default will force the form validator to display error messages if a value is not provided.
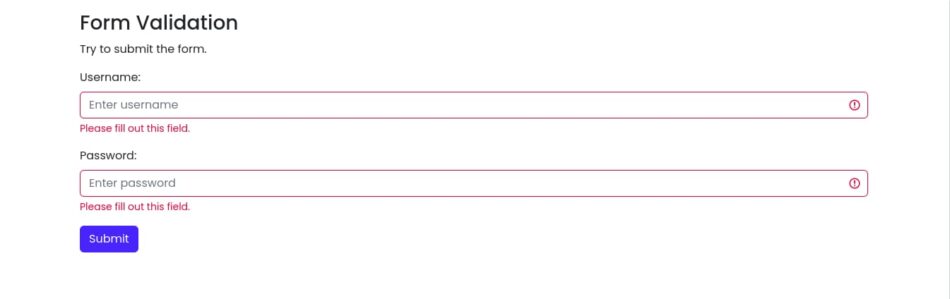
You do not need to add
blank=False
in your model field. Django set the value by default.
How do null=True
and blank=True
affect database migrations in Django?
When dealing with null
and blank
model field options in Django, the database columns may accept NULL values at any one point. Sometimes, you may want to change for the database to accept values by removing null
field option.
In such cases, you will have to migrate for changes to reflect in your database. When you do that, Django does not change the values of the objects that were previously stored.
Here is what I mean:
Let’s say you had previously set null=True
and now you want to change to null=False
(to default).
After changing the field option, any object created will need to have a value from that moment. However, the previously created objects will have NULL values.
Django will prompt you to provide a default value for the previously created objects if you run your migrations.
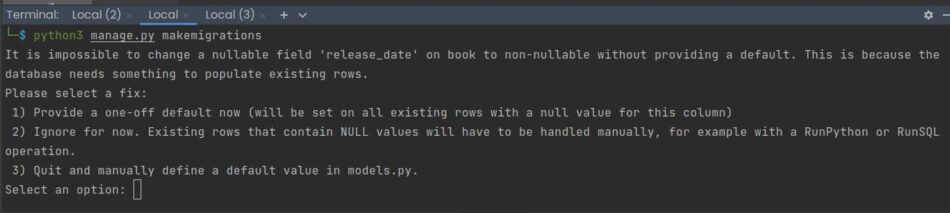
In that case, you need to:
- Explicitly define the default value to be tabulated for the existing objects OR
- Provide an extra model field option,
default
that allows you to declare the value to be tabulated in the existing objects.
If you run your migrations, Django will not have a problem. The already created objects will now have the default value you specified in their DB table columns.
On the other hand, when you change null=
False to null=True
, Django will not populate the field’s value as NULL for any existing object. Django will only change the column definition to allow NULL values.
Any existing objects will have the value that was previously stored.
NOTE: Changing the value of
null
to True for a database with existing records can have an impact on the structure and integrity of data in the following ways:
- Data loss for objects that had previous data that gets overridden with the default values or NULL. If the previous data was useful, such data will end up being lost.
- Inconsistency when default values do not match existing data. The database columns will end up storing NULL, empty, or other consistent values across a few objects. Such a database can have serious problems when querying or analyzing the data.
- You may have constraint exceptions when other fields or tables depend on the field that was previously holding some value and now can accept NULL or empty values.
Is it necessary to set both null=True
and blank=True
in a Django model field?
Specifying null=True
and blank=True
in any Django model field depends on the specific use case of the field and the data integrity you wish to maintain.
The setting null=True
allows a field to store NULL values in the database. blank=True
field option allows a field to have empty values when filling a form.
When you are not performing any custom validation when creating or updating an object, it is a good practice to set both null=True
and blank=True
. This allows a field to be left empty during form validation and avoids errors that may arise during database validation when creating or updating an object.
Suppose you are overriding the save method to explicitly set the field’s value before saving the object, or you have explicitly defined the default value. In that case, you should not include null=True model field option. In that case, you should set blank=True only. An example would be when you need to set an article’s author as the currently logged-in user. You do not need the user to type in the username in the form.
Tip
You should avoid using null=True
for string-based fields such as CharField
and TextField
. The reason is to avoid having data redundancy where the database columns will have two possible values: None or an empty string.
As a Django convention, for CharField and TextField, you should only use blank=True
class Person(models.Model):
...
address = models.TextField(max_length=500, blank=True)