Can I use Bootstrap 5 with Django?
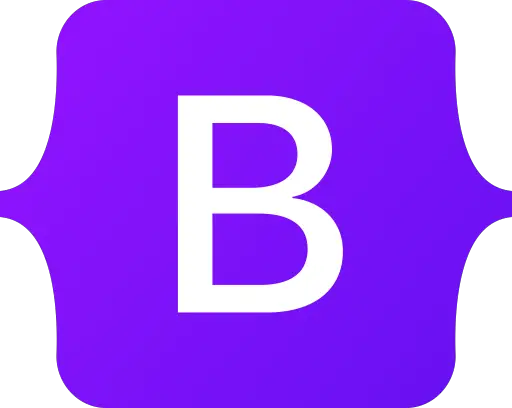
When it comes to building responsive Django web applications, Bootstrap is the best CSS framework that you can use to style your pages easily. Thus, achieving your rapid development objectives for your web development project, as a web developer. Bootstrap keeps on updating, and you would wish to use the current Bootstrap 5 version in your Django project. Thus, you want to know if you can use Bootstrap 5 with Django.
You can use Bootstrap 5 with your Django project as the preferred front-end CSS framework. Bootstrap provides already-built CSS that helps you style your Django web pages and JavaScript components that help you create buttons, forms, and navigation.
The good thing is that you do not have to struggle to add Bootstrap 5 to your Django project. You can easily add Bootstrap 5 following these three approaches:
- Manually downloading and including Bootstrap 5 files in your static files
- Using a Content Delivery Network (CDN)
- Using
django-bootstrap5
PyPI package
Manual Integration of Bootstrap 5 in Django: Downloading and including files in the static folder
Here are the steps needed to manually download Bootstrap 5 files into your Django project static files directory:
Step 1: Download Bootstrap 5 files from the official website
Head over to the official Bootstrap website and download the latest compiled CSS and JS files.
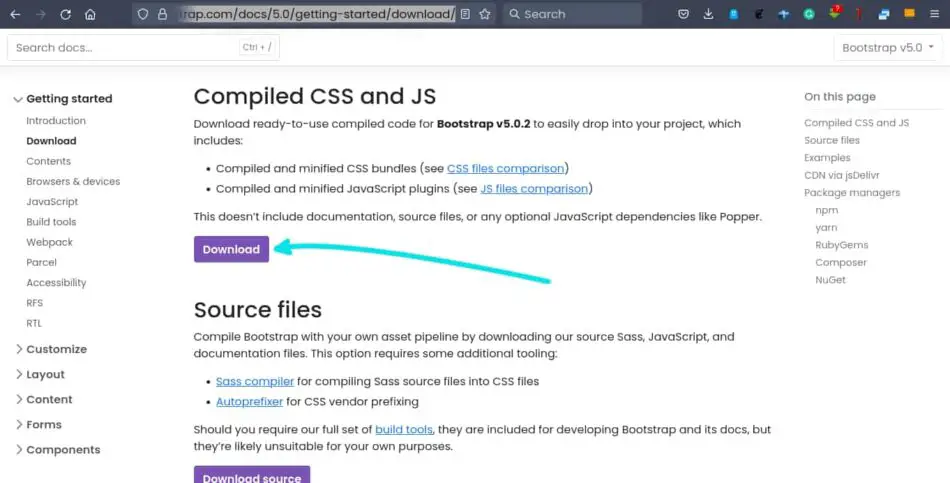
Step 2: Create static files directory (if you do not have one yet)
Inside your Django project root directory (where you have your manage.py file), create the static files directory, if you haven’t created it yet. Name the directory ‘static’.
mkdir static
You will use this directory to store all your Bootstrap 5 CSS and JS files; and your custom CSS and JS files.
Step 3: Add Bootstrap 5 files to the static directory
After creating the static
directory, extract the Bootstrap 5 CSS and JS files inside this directory. In the downloaded Bootstrap 5 .zip file, extract only the CSS and JS directories.
Step 4: Update your settings.py file to include static
path directory
Open your main project settings.py
file and make sure you have the STATICFILES_DIRS
setting. Besides, the STATICFILES_DIRS
should include the path to the static files directory we created.
The setting instructs Django to find the static files (CSS, JS, and image files) in that directory.
Add the following line to your settings.py file:
STATICFILES_DIRS = [BASE_DIR / 'static',]
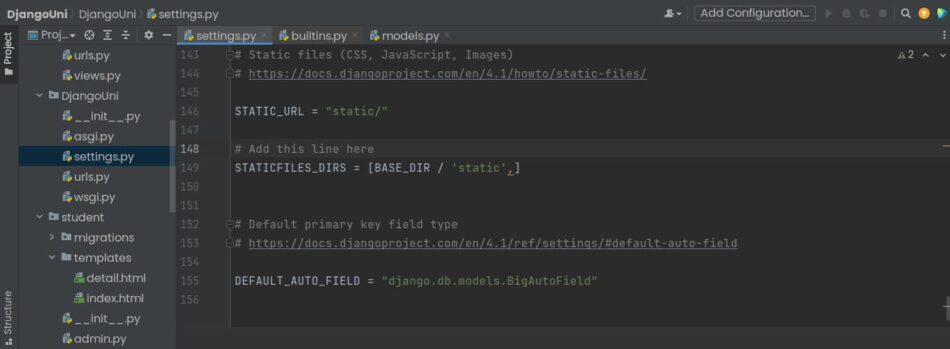
The setting, STATIC_URL
is set to /static/
, which will be the URL that will be used to access the static files used in your Django project.
The second setting, STATICFILES_DIRS = [BASE_DIR / 'static',]
, is set to include the static
directory we created earlier. The setting tells Django to look for static files in the directories listed here.
BASE_DIR
is a built-in variable in Django that represents the root directory of the project.
Step 5: Link Bootstrap 5 files in your BASE.html file
When using Bootstrap in your Django project, it is best practice to load the CSS and JS files in one HTML file and create blocks that child HTML templates will inherit from the base template file. Learn more about template inheritance in this article.
So, create base.html inside your templates directory
touch templates/base.html
Be sure to instruct Django to look for template files in this directory by having the following setting in your settings.py file:
TEMPLATES = [
{
"BACKEND": "django.template.backends.django.DjangoTemplates",
# Instruct Django to include project-level templates directory
"DIRS": [BASE_DIR / 'templates'],
"APP_DIRS": True,
"OPTIONS": {
"context_processors": [
"django.template.context_processors.debug",
"django.template.context_processors.request",
"django.contrib.auth.context_processors.auth",
"django.contrib.messages.context_processors.messages",
],
},
},
]
In the head section of your base.html file, add the following code that links Bootstrap 5 CSS and JS minified files.
{% load static %}
<!-- base.html -->
<head>
<link rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}">
<script src="{% static 'js/bootstrap.min.js' %}"></script>
</head>
With that, you can use Bootstrap 5 classes in your base.html files.
<!-- base.html -->
<body>
<div class="container-fluid bg-primary text-white py-3">
<h1 class="text-center">My Django Website</h1>
</div>
</body>
To use Bootstrap 5 classes and components, create blocks that child templates will use. A common block is the block content that allows you to add body content to your .html child templates.
In your base.html file, add the following code inside the <body> tag:
<body>
<div class="container-fluid bg-primary text-white py-3">
<h1 class="text-center">My Website</h1>
</div>
<div class="container-fluid">
{% block content %}
{% endblock %}
</div>
</body>
Step 6: Use Bootstrap 5 classes and components in your .html files
Every time you create a new .html file, you can use Bootstrap 5 classes and components in these templates by using the block we created earlier to inherit parent styling including Bootstrap 5.
Here’s an example:
{% extends "base.html" %}
{% block content %}
<div class="row">
<div class="col-md-8 offset-md-2">
<---- your html code goes here ----->
</div>
</div>
{% endblock %}
Integrating Bootstrap 5 with Django using a Content Delivery Network (CDN)
The second approach of using Bootstrap 5 with Django involves using a CDN. Here are the steps you need to take to integrate your Django project with Bootstrap:
Step 1: Get the Bootstrap 5 CDN links
Head over to Bootstrap 5 official website and copy the CDN links offered by jsDelivr.
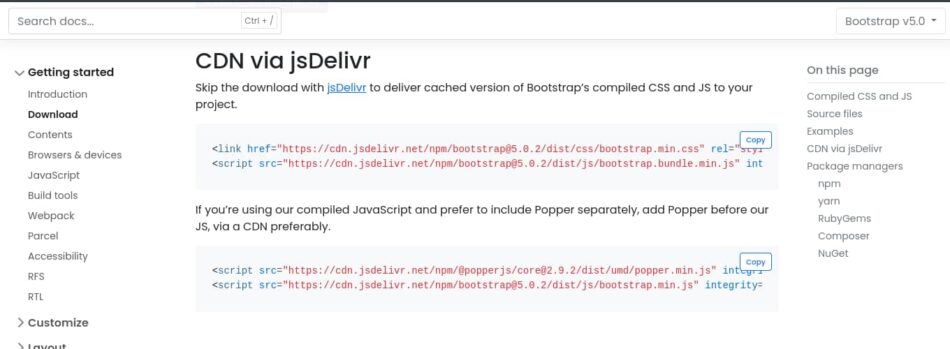
Step 2: Add the links to your base.html file
In the head section of your base.html file, add the following code that links Bootstrap 5 CSS and JS minified files CDNs.
<head>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
</head>
Step 3: Use Bootstrap 5 classes and components
You can start using Bootstrap 5 classes and components in your templates by using the blocks to inherit the parent (base.html) styling including Bootstrap 5 styles.
{% extends "base.html" %}
{% block content %}
<div class="row">
<div class="col-md-8 offset-md-2">
<---- your html code goes here ----->
</div>
</div>
{% endblock %}
django-bootstrap5: A Simple and Efficient Way to Incorporate Bootstrap 5 in your Django Project
The third approach to using Bootstrap 5 in your Django project is to use a third-party package called django-bootstrap5. The package allows you to include Bootstrap 5 in your Django project using template tags and widgets.
It simply removes the complexities of manually adding Bootstrap 5 to your Django project.
The best way to use bootstrap in Django is to use the django-bootstrap5 package which saves you time, effort, and complexities of including Django in your project and leveraging its features.
For this approach, check out this dedicated guide where I show you how to use Bootstrap 5 the right way while including an example project.