How to use elif conditional in Django Templates
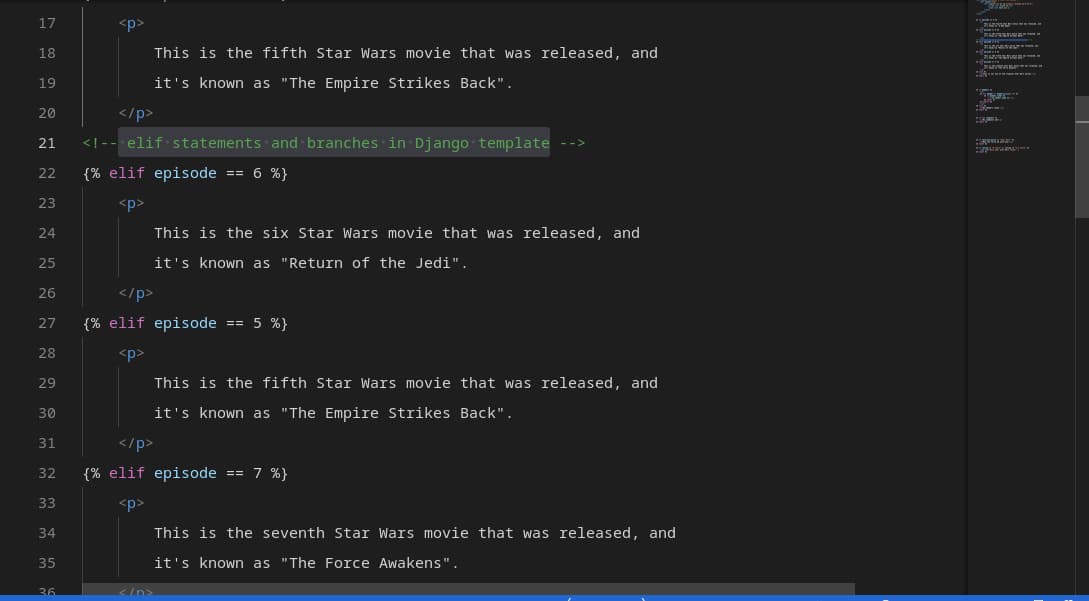
While the {% if %} and {% else %} tags are the most commonly used conditional statements in Django templates, the {% elif %} tag provides a powerful tool for handling more complex scenarios.
We’ll take a closer look at how to use elif conditionals in Django templates, including syntax, examples, and best practices.
If you’re new to conditional statements in Django templates, you may want to check out this Ultimate Guide on how to use if else conditions in Django templates.
Let’s start by answering,
Can we use elif in Django template?
You can use the “elif” conditional statement in Django templates to provide additional conditions to an “if” statement, allowing for more complex and dynamic control flows in your Django templates.
By using “elif” statements in your templates, you can create more sophisticated logic that responds to various scenarios and input data.
How to use elif in Django templates
In addition to the basic “if” and “else” statements, the Django template language also supports the use of the “elif” statement.
With “elif,” you can add additional conditions to your “if” statements, allowing for more complex and flexible logic.
Let’s explore if…elif…else conditional syntax, and examples of use cases.
Syntax of if elif else condition in Django templates
The syntax of if-elif-else conditionals in Django templates is very similar to that of standard programming languages like Python.
The basic structure includes
- An opening {% if %} tag, followed by
- One or more {% elif %} clauses, and finally
- An optional {% else %} tag to catch any remaining conditions.
Here are the key elements and tags used in the syntax:
- {% if %} tag: This is the opening tag for the if statement and specifies the initial condition to be evaluated.
- {% elif %} clause: This tag is used to add additional conditions to the if statement. You can include multiple elif clauses as needed.
- Elif branches: These are the individual code blocks associated with each elif clause, and they contain the code that should be executed if the corresponding condition is met.
- {% else %} tag: This tag is optional and specifies what code to execute if none of the previous conditions are met. If you include an else tag, it should be placed at the end of the if-elif-else block.
- {% endif %} tag: This is the closing tag for the if statement.
To use these elements in your Django templates, you simply need to follow the standard syntax for if…elif…else statements.
Here is an example of the basic syntax for an if-elif-else block in a Django template:
{% if some_variable == 'foo' %}
<p>The variable is equal to foo</p>
{% elif some_variable == 'bar' %}
<p>The variable is equal to bar</p>
{% else %}
<p>The variable is something else</p>
{% endif %}
Examples of basic if-elif-else statements in Django templates
Here are a few examples of basic if-elif-else statements in Django templates that demonstrate how to use elif syntax in real-world scenarios:
Example 1: Display unique content based on user login status
Suppose you have a website that requires users to log in. You want to display different content based on their status (logged in, not logged in, etc.).
You can use an if-elif-else block to achieve this by using some code implementation that looks like this:
{% if user.is_authenticated %}
<p>Welcome back, {{ user.username }}!</p>
{% elif request.path == '/login/' %}
<p>Please log in to access this page.</p>
<!---- you may provide a login link here---->
{% else %}
<p>You must be logged in to access this page.</p>
<!---- you may redirect a user to a page where they are allowed to access---->
{% endif %}
In this example, the first condition checks whether the user is authenticated, i.e. logged in. If so, it displays a personalized welcome message.
If the user is not logged in, the elif clause checks whether the current page is the login page. If so, it displays a message asking the user to log in.
If neither of these conditions is met, the else clause displays a generic message asking the user to log in.
Example 2: Provide different information based on product availability in an e-commerce store
Suppose you’re creating an e-commerce site that sells products, and you want to display different content based on their availability.
You can use an if-elif-else block to achieve this:
{% if product.stock > 0 %}
<p>This product is in stock and ready to ship.</p>
{% elif product.preorder %}
<p>This product is available for preorder.</p>
{% else %}
<p>This product is currently out of stock.</p>
{% endif %}
In this example, the first condition checks whether the product is currently in stock, and if so, it displays a message indicating that it’s ready to ship.
If the product is not in stock, the elif clause checks whether it’s available for preorder. If so, it displays a message indicating that the user can preorder it.
If neither of these conditions is met, the else clause displays a message indicating that the product is out of stock.
How to combine if…elif…else with other Django template tags
To combine if...elif...else
statements with other Django template tags, you simply need to nest the tags inside one another.
This allows you to create more complex logic and dynamic content in your templates.
Here’s an example:
Suppose you have a website with different sections, and you want to display a different header image for each section.
You can use the
include
tag to include a separate template file for each section, and anif...elif...else
block to determine which template to include:{% if section == 'home' %} {% include 'home_header.html' %} {% elif section == 'about' %} {% include 'about_header.html' %} {% else %} {% include 'default_header.html' %} {% endif %}
As you can see, combining if...elif...else
conditional statements with other Django template tags is a powerful way to create dynamic and responsive websites.
By using these techniques, you can create templates that adjust their content based on a wide range of variables and user input.
Difference between if else and if elif in Python Django
In Django template system, if...else
and if...elif...else
are two conditional statements that you can use to create more complex logic and control the content displayed in your templates.
The if...else
statement allows you to execute one block of code if a condition is true, and another block of code if it is false.
The if...elif...else
statement, on the other hand, allows you to check multiple conditions and execute different code blocks based on the results.
To understand the differences between these two statements in more detail, take a look at the table below:
if...else Statement | if...elif...else Statement |
---|---|
Evaluates a single condition | Evaluates multiple conditions |
Only one block is executed | Only one block is executed based on the first true condition, even if others are also true |
Used for simple boolean checks | Used for complex decision-making branches and conditions |
Example syntax used:{% if condition %} | Example syntax used: `{% if condition %} Execute this block {% elif %} Execute this block if previous checks are false {% elif %} Execute this block if previous checks are false {% endif %} |
Best practices for using if-elif-else in Django Templates
There are some best practices that you should follow to ensure that your code is efficient, maintainable, and easy to understand.
- Use the
if
statement for simple checks: If you only need to perform a simple boolean check, use theif
statement instead of the more complexif...elif...else
statement. - Keep your code readable: Use whitespace and indentation to make your code more readable. This will make it easier to understand your code, especially if you are working with complex
if...elif...else
statements. - Use
elif
to handle multiple conditions: If you have multiple conditions to check, use theelif
statement to simplify your code and make it easier to read. - Use
else
for default cases: Use theelse
statement to handle default cases, where none of the other conditions are true. - Avoid nesting too many
elif
branches: Nesting too manyif
statements can make your code difficult to read and understand. Instead, consider refactoring your code to use more conciseif...elif...else
statements. - Use boolean operators: You can simplify your code by using boolean operators such as
and
andor
to combine conditions.