Create a for loop with if condition in Django templates
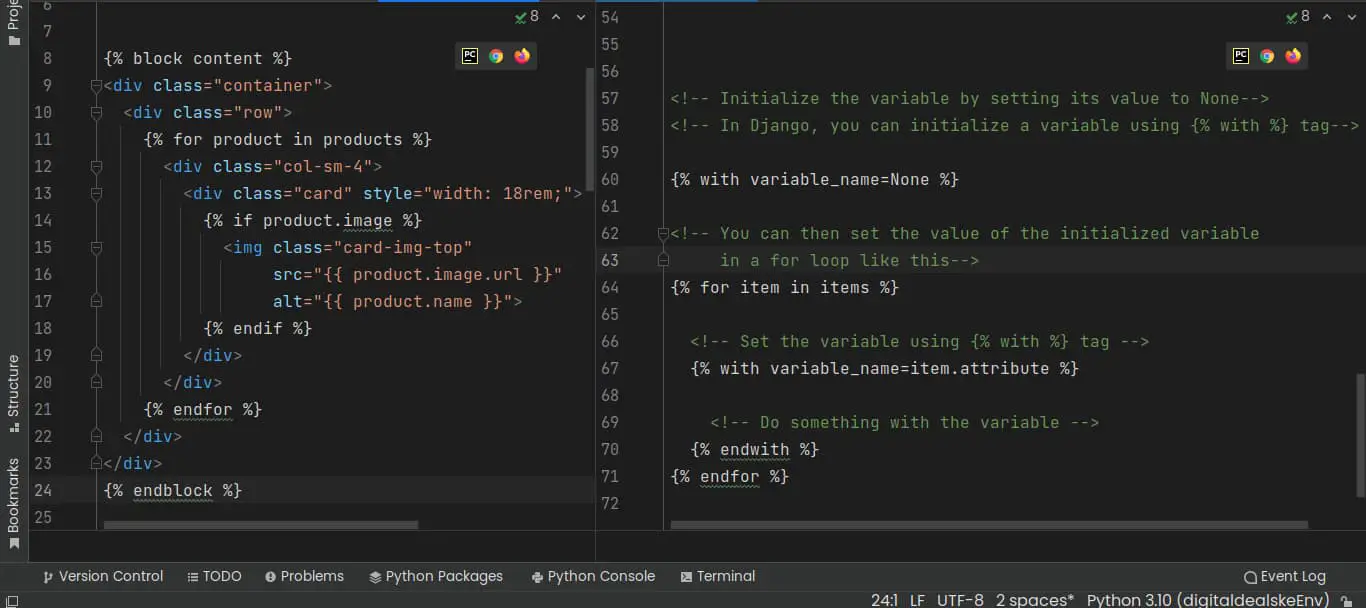
In almost any of the projects that you’re going to develop as a developer, you are going to iterate through a list of objects and selectively choose which objects to display to the users. I find Django templates to be very powerful when creating customizable and dynamic HTML pages.
One of the features that I have mostly used in Django templates is the ability to use a for loop with an if condition allowing me to iterate through a list of objects and display specific objects items selectively.
In this article, I will show you how to use a for loop with an if condition to create a more dynamic display of your data on web pages.
But first, can we do that?
Is it possible?
Can I put an if statement in a for loop?
You can insert an if else statement in a for loop tag when dealing with Django templates. Putting an if condition in a for loop allows you to loop through a list of items or objects and only display specific items/objects that satisfy the condition.
Why use a for loop with an if condition?
Using an if condition in a for loop is a useful feature in Django templates that allows you to:
- Create more dynamic web pages
- Create efficient and effective templates that can adapt to changing data and user input
- Improve web application user experience by making your web pages more engaging and interactive for your audience
Here are several reasons why you might want to use a for loop with an if condition in Django templates.
- Display data based on conditions or criteria
- Create more dynamic content that can adapt to changing data from the for loop
- Write more efficient code that only displays data that is necessary and important to a user
- Create personalized content based on the data a user may provide
How to create a for loop in Django templates
With a for loop, you can be able to iterate through a list of data or objects from the database and display them in a formatted and ordered way.
Here are the steps you need to create an effective for loop in Django templates:
- Define the context in your views before creating a for loop in Django templates
- Start the for loop in your Django templates by using the correct template tags,
{% for %}
and{% endfor %}
- Note the context variable defined in your view and supply it to the {% for %} template tag as an argument.
- Display individual data from each iteration using the variable specified in the for loop tag. You can wrap the data in a standard HTML tag or Django template tag. You can use dot notation to access the attributes and properties of an object.
- If you have a nested data structure, such as a list of lists, you can use nested loops to iterate through all levels of the data.
Here are the steps in detail and with elaborate examples:
How to iterate over a list of objects or items using a for loop in Django templates
Step 1: Define the context in your view
In views.py
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
# Defined the context variable 'products'
# I will use to iterate and get a list of products
context = {'products': products}
return render(request, 'product_list.html', context)
Step 2: In your template file, create a basic {% for %} loop template tag
{% extends 'base.html' %}
{% block content %}
<div class="container">
<div class="row">
{% for %}
{% endfor %}
</div>
</div>
{% endblock %}
Here, I am declaring a for loop template tag and wrapping it in a Bootstrap div.
Step 3: Supply the context as an argument to the {% for %} template tag
Inside the {% for %} tag, you have the ability to supply the context variable we declared in our views as an argument using the syntax: '{% for your_context_variable_name %}
{% extends 'base.html' %}
{% block content %}
<div class="container">
<div class="row">
{% for product in products %}
{% endfor %}
</div>
</div>
{% endblock %}
Step 4: Display data of each iteration in a normal HTML tag or other Django template tags
{% extends 'base.html' %}
{% block content %}
<div class="container">
<div class="row">
{% for product in products %}
<div class="col-sm-4">
<div class="card" style="width: 18rem;">
<div class="card-body">
<h5 class="card-title">{{ product.name }}</h5>
<p class="card-text">{{ product.description }}</p>
</div>
</div>
</div>
{% endfor %}
</div>
</div>
{% endblock %}
Here, I iterate through a list of products and once each product is retrieved, I display each iterated product’s name and description in a <h5> and <p> HTML tags respectively.
Step 5: Create a nested for loop if you have a nested data structure
Suppose you have a list of orders, where each order contains a list of products. To display the products in each order, you can use a nested for loop like this:
{% for order in orders %}
<h2>Order #{{ order.id }}</h2>
<ul>
{% for item in order.items.all %}
<li>{{ item.name }} - {{ item.price }}</li>
{% endfor %}
</ul>
{% endfor %}
In this example, I am using a nested for loop (a for loop within a for loop) to access the orders and in each order access the list of items in that order.
How to add an if condition to a for loop in Django templates
To add an if
condition to a for loop in Django templates, you can use the {% if %}
template tag within the for loop template tag, {% for %}.
Just like with a nested for loop, you can create an {% if %} tag within a for loop to selectively display or process data based on criteria or condition. The {% if %}
tag is used to check a condition and execute the code within the block if the condition is true.
Once you have defined the if, elif, and else conditions, you can use Django template tags and HTML tags to display the data within the block if the condition is satisfied.
To write an elif statement in Django templates, use {% elif %}
syntax.
To write an else statement in Django templates, use {% else %}
syntax.
The basic syntax of an if condition in templates
The basic syntax of an if
condition in Django templates is as follows:
{% if condition %}
code to execute if condition is true
{% endif %}
In this syntax, {% if condition %}
starts the if
block, where condition
is the Python expression to be evaluated. If the condition is true, the code within the block is executed.
The {% endif %}
tag is used to close the if
block.
The basic syntax of an if
condition with elif and else conditions in Django templates is as follows:
{% if condition_1 %}
code to execute if condition 1 is true
{% elif condition_2 %}
code to execute if condition 2 is true
{% else %}
code to execute if all conditions are false
{% endif %}
Using if conditions in conjunction with a for loop
You can use if conditions in conjunction with a for loop in Django templates to filter or select specific data from a collection of objects, and then perform some action on the selected data.
The basic idea is to use an if
condition within the for loop to check whether each item in the collection meets a certain criterion, and then only process or display the items that meet that criterion.
In a real-world example, you can use a for loop to iterate over a list of products. If you only want to display products that are within a price range, you can use an if
condition within the for
loop to only display the products that are within that price range.
In another example, you can retrieve a list of blogs and within that list, display only the blog posts that are published. Besides, you filter to retrieve blog posts only if they meet a certain word length or comments.
The basic syntax for using an if
condition with a for
loop is as follows:
{% for item in items %}
{% if condition %}
code to execute if condition is true
{% endif %}
{% endfor %}
In this syntax, {% for item in items %}
starts the for loop, where item
is the name of the loop variable and items
is the collection of objects to iterate over.
Inside the for loop, I created an {% if condition %}
to check whether the current item meets a certain condition. If the condition is true, the code within the if block is executed.
The {% endif %}
tag is used to close the if
block.
The {% endfor %}
tag is used to close the for loop.
Example for loop with if condition in Django templates
Here’s an example of a for loop with an if condition in Django templates from a real-world scenario that you could be implementing for your Django project:
Let’s say you are building an e-commerce website that sells products in different categories. You want to display the list of products that belongs in a specific category on a specific page.
Assuming you have the list of products and the selected category passed to the template as context variables, you can use a for loop with if condition to filter the products based on the selected category.
Here is an example code implementation:
<!-- Iterate through all your products in the db by defining the for block -->
{% for product in products %}
<!-- Check whether the current product belongs to the selected category-->
<!-- products.categories.all is the reverse relationship to the categories that the product belongs to-->
{% if selected_category in product.categories.all %}
<!-- If the product is in the selected category, display its name, description, and price-->
<div class="product">
<h2>{{ product.name }}</h2>
<p>{{ product.description }}</p>
<p>Price: {{ product.price }}</p>
</div>
<!-- Close the if block-->
{% endif %}
<!-- Close the for block-->
{% endfor %}
How to handle empty lists or objects in a for loop with an if condition
When working with a for loop, it’s important to handle cases where the list or object being iterated over is empty. Otherwise, the loop will not execute, and the page may not display the desired content.
To handle exceptions that may arise from dealing with empty lists, you can use a for loop within an if statement like this:
{% if products %}
{% for product in products %}
<div class="product">
<h2>{{ product.name }}</h2>
<p>{{ product.description }}</p>
<p>Price: {{ product.price }}</p>
</div>
{% endfor %}
{% else %}
<p>There are no products yet.</p>
{% endif %}
In this example, {% if products %}
tag checks if the products
is not empty. If it is not empty, the for
loop inside the if
block will iterate through the list of products and display the product’s name, description, and price.
However, if products
context is empty, the code inside the else
block will execute and display a message that there are no products in the store yet.
By handling empty data successfully, you, as a web developer, are able to achieve the best practices for developing web applications, which are:
Best practices for handling empty data
- Always checking for empty data before attempting to use it on your web pages. The practice helps avoid errors or any other unexpected behavior that may ruin user experience.
- Providing informative feedback if a user experiences a scenario where there is no data in the database.
- Avoiding empty elements on a page, which make a page look cluttered or confusing.
- Providing default values to create a more consistent user experience and expectations. A good example is where an image is expected. But when an image is unavailable, you should collapse the space. Instead, provide a default image to maintain page layout consistency.
How to set a variable in a for loop in Django templates
You can set variables within a for loop using the {% with %}
template tag in Django. The {% with %}
tag allows you to define a new variable within the current template context. The approach is important when you want to create a variable that you can reuse multiple times in that template.
Here’s the basic syntax for using the {% with %}
tag to set a variable within a for loop in Django templates:
<!-- Initialize the variable by setting its value to None-->
<!-- In Django, you can initialize a variable using {% with %} tag-->
{% with variable_name=None %}
<!-- You can then set the value of the initialized variable in a for loop like this-->
{% for item in items %}
<!-- Set the variable using {% with %} tag -->
{% with variable_name=item.attribute %}
<!-- Do something with the variable -->
{% endwith %}
{% endfor %}
Basically, to set a variable in a for loop in Django templates, follow these steps:
- Initialize the variable by setting its value to None using
{% with my_variable=None %}
syntax - Define your for loop using the
{% for item in items %}
syntax - Within your for loop, set the value of the variable using the
{% with my_variable=item.attribute %}
syntax - Perform some operations with the new variable referencing your iterable attribute.