What is the difference between .get() and .filter() methods in Django?
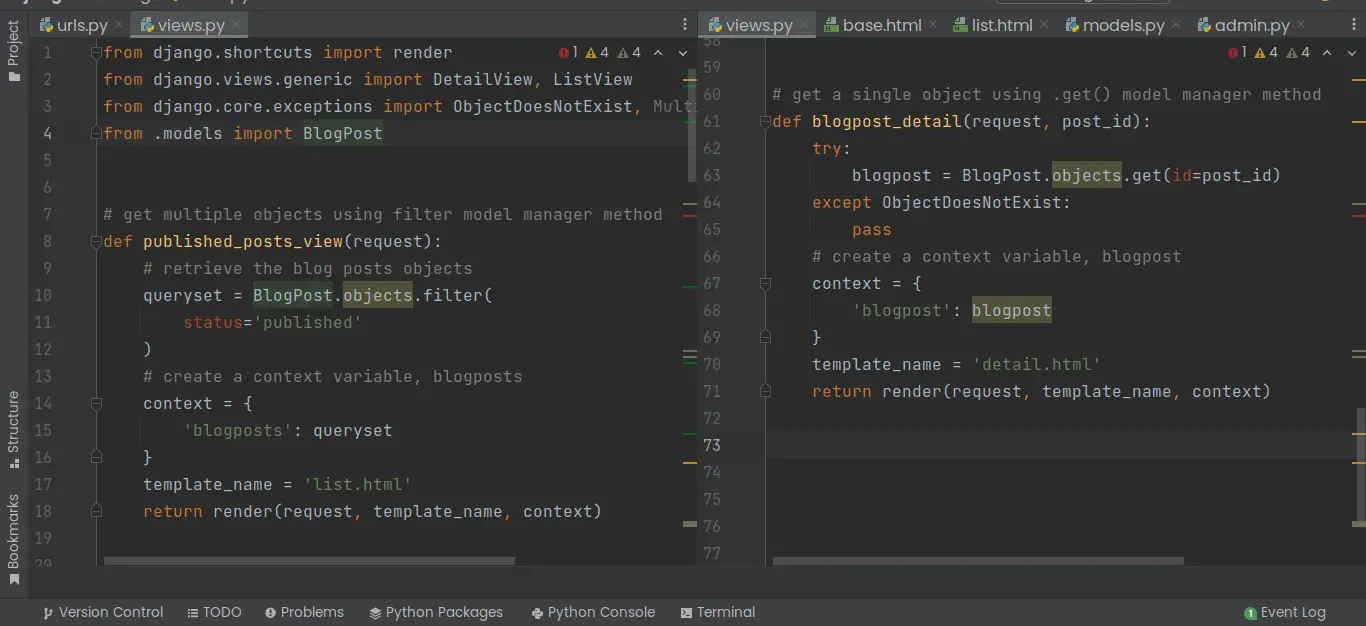
You have written your Django models. You want a way to retrieve the data from the database and probably display it to the users or create API endpoints.
To achieve that, you must retrieve the object from the database, write a view or serializer to send the data to a template or API endpoint, and then create a template or API that will render the object’s data on a web page.
You can get a single object using the .get() model manager method or retrieve one or more objects using the .filter() model manager method. Here are the differences between the get and filter methods in Django:
objects.get() method objects.filter() method Used to retrieve one item from the Model table. Used to retrieve one or more items from the Model table. Queries are never lazy loaded. Thus, queryset objects are not created when retrieving a single object. There are instances when queries are lazy loaded. When retrieving more than one item, queryset objects are created and lazy loaded. The queries in the queryset object are only evaluated when converted into a list or iterated over to get each object. You must be sure there is only one unique entry in the database. Returns multiple items based on the specified field lookup value. Exploits primary keys or ids as unique identifiers for every single item in the database. Exploits field lookup values similar across multiple items in the database You cannot chain multiple get() methods together. You can chain filter() methods together. Example: Model.objects.get(pk=1) will return a single item from the Model table with the id of 1. Example: Model.objects.filter(pk=1) will return a single item from the Model table with the id of 1.Model.objects.filter(status=’published’) will return multiple items that match the published status.
So, the process of retrieving one or more objects in Django looks like this:
Identify the field lookup values
With the field lookup values, you can specify whether you wish to retrieve one or more objects.
Retrieve the object’s data using the field lookup values
Depending on whether a field lookup value is unique to each object or not, you will get single or multiple objects.
A single object is returned when you use a field lookup value unique to a particular object.
A field lookup value that exists across multiple objects will return multiple objects.
Create your queryset in function-based or class-based views
After figuring out whether you wish to retrieve a single object or multiple of them, create the code for retrieving the objects in a view.
Associate your view to a URL endpoint
Create a URL endpoint for users to access the objects retrieved from the database. Example: create the /blog/ URL for users to access all the blog post objects on a web page.
Besides, /blog/1/ or /blog/slug-value-here/ to access a single object.
To retrieve data or objects from the database, you have the choice of using two functions:
- Model.objects.get() and
- Model.objects.filter() methods.
These two methods provide different functionality that we will see in a couple of minutes.
Before we do that, we must understand how we retrieve objects stored in the database.
You can retrieve a single object or a couple of objects. Besides, you must tell/specify to Django what kind of object you want to retrieve from the database.
What attributes, rows, and columns to target when retrieving data?
Thus, we must have the functionality to retrieve a single object that matches a certain keyword/value or multiple objects that match a particular keyword or field value.
In simple terms, the process of matching an object or multiple objects with a certain keyword and returning only those objects is called querying.
Now back to get() and filter() methods.
The get() and filter() methods are most commonly used when querying the database for data stored in objects. The .get() and filter() methods are called model manager methods as they are accessed using the default Django model manager, objects.
You can get one or more objects from the database with the get() method and filter() methods, respectively.
The .get() model manager method is used to query the database to retrieve a single object.
The .filter() method can also retrieve a single object by using a field lookup value that is unique to a particular object.
Besides, you can use the .filter() method to get more than one object from the database.
How to get a single item using the .get() method in Django
The get() method retrieves only single objects stored in a Django database using a unique query that only matches one instance of an object. Django will throw an error indicating multiple objects returned instead of a single object if the query matches multiple objects.
Thus, it would be best to use a unique query that matches only one single object stored in the database.
So, an example would be to use an id of an object to retrieve a particular object that matches an existing id in the id column table.
Model.objects.get(id=1)
Also, you can use other fields declared in your Model attributes to retrieve items in the database.
For example, if I am sure only one person has a unique last name, I can use the last_name field to query the database for this particular person.
Note, if two or more persons have the same last name, using the get() method will not work– Django will throw an exceptions
Model.objects.get(last_name=”Nganga”)
Again, be careful not to use a query filter that would match multiple objects. An example where a MultipleObjectsReturned error would be returned is when you get an object using the last name, first name, or surname similar to more than one person.
Let’s say you have these students in your database:
- Leia Skywalker
- Anakin Skywalker
- Rey Skywalker
If you want to get Anakin Skywalker using the last_name attribute, you will use the get() method like this:
Jedi.objects.get(last_name=’Skywalker’)
However, if you execute the code above, Django will throw an exception because it encounters multiple rows/Jedi matching the last_name, Skywalker.
How to handle exceptions associated with the .get() method when retrieving an object
1. Be sure the item you are getting from the database exists.
Thus, you must know the value of the attribute you are using to look for the object in your database table. Otherwise, when you do not know the exact value, you can use alternative approaches, such as wrapping the .get() method in an exception block or using the .filter() method.
2. Use a try … except block to catch ObjectDoesNotExist and MultipleObjectsReturned exceptions
You may use an exception block to prevent Django from throwing exceptions, such as ObjectDoesNotExist and MultipleObjectsReturned. Here’s how you do that:
Import the exception handler first –
from django.core.exceptions import ObjectDoesNotExist, MultipleObjectsReturned
Then use a try … catch block to handle the exceptions once encountered
try:
item = Model.objects.get(id=44849)
except ObjectDoesNotExist:
define the error you want to show to the user e.g. indicate the object could not be found.
except MultipleObjectsReturned:
Alert the use there are multiple objects returned
When using the .get() method, Django sends the queries to the database without having to store them in a queryset object.
A queryset is an object that contains a query and a set of queries. Django will then evaluate the queryset to generate the right SQL statements that are sent to the database and retrieve the items.
In this article, you can read more about how to get a single object from the database in Django.
How to get multiple items using objects.filter() method in Django
The .filter() model manager method is used to query the database returning one or more items that match a particular field lookup value. The lookup keyword can be in more than one object. Therefore, Django filters out the objects that do not contain the keyword used in the query and only return objects matching the query.
So, when using the filter() method, you can access one object by using a field lookup value that is unique to a particular object.
For example, using the id field is a conventional approach to getting one item from the database in Django.
Alternatively, you can use the .filter() method to get multiple objects from the database. When using the .filter() to get multiple objects, the queries are lazy loaded.
Here, you can use a field lookup value that matches more than one object, such as a ForeignKey or any other field where its value can be in multiple objects.
For example, with the ForeignKey, you can retrieve all the objects that match a particular ForeignKey field value. You can retrieve multiple blog posts or products that are in one category. So, you use the category field defined in your BlogPost or Product model class and the category id in your filter method. Thus, returning all the blog posts and products in that category.
query_set = BlogPost.objects.filter(category__id=1)
The code above returns multiple blog post objects in the category, Finance, which has an id of 1.
The same for the Product model
query_set = Product.objects.filter(product_category__id=2)
When using the .filter() method to target multiple objects, Django sends the queries to the database while lazy loading the queries.
Thus, Django stores the queries in a queryset object that only gets evaluated when you loop through the queryset to get each object or convert the queryset into a list.
A queryset is an object that contains a query and a set of queries. Django will then evaluate the queryset to generate the right SQL statements that are sent to the database and retrieve the items.
In this article, you can read more about how to get multiple objects from the database in Django.
In summary
Differences between Model.objects.get() and Model.objects.filter() methods
objects.get() method | objects.filter() method |
---|---|
Used to retrieve one item from the Model table. | Used to retrieve one or more items from the Model table. |
Queries are never lazy loaded. Thus, queryset objects are not created when retrieving a single object. | There are instances when queries are lazy loaded. When retrieving more than one item, queryset objects are created and lazy loaded. The queries in the queryset object are only evaluated when converted into a list or iterated over to get each object. |
You must be sure there is only one unique entry in the database. | Returns multiple items based on the specified conditions. |
Exploits primary keys or ids as unique identifiers for every single item in the database. | Exploits attribute values similar across multiple items in the database |
You cannot chain multiple get() methods together. | You can chain filter() methods together. |
Example: Model.objects.get(pk=1) will return a single item from the Model table with the id of 1. | Example: Model.objects.filter(pk=1) will return a single item from the Model table with the id of 1.Model.objects.filter(status=’published’) will return multiple items that match the published status. |
Django associates the primary key to the automatically created id of an object or item. Thus, each data table entry will then having a unique entry each having an additional id field that is used as the primary key.